SWIFT programming language - what's behind it?
Published
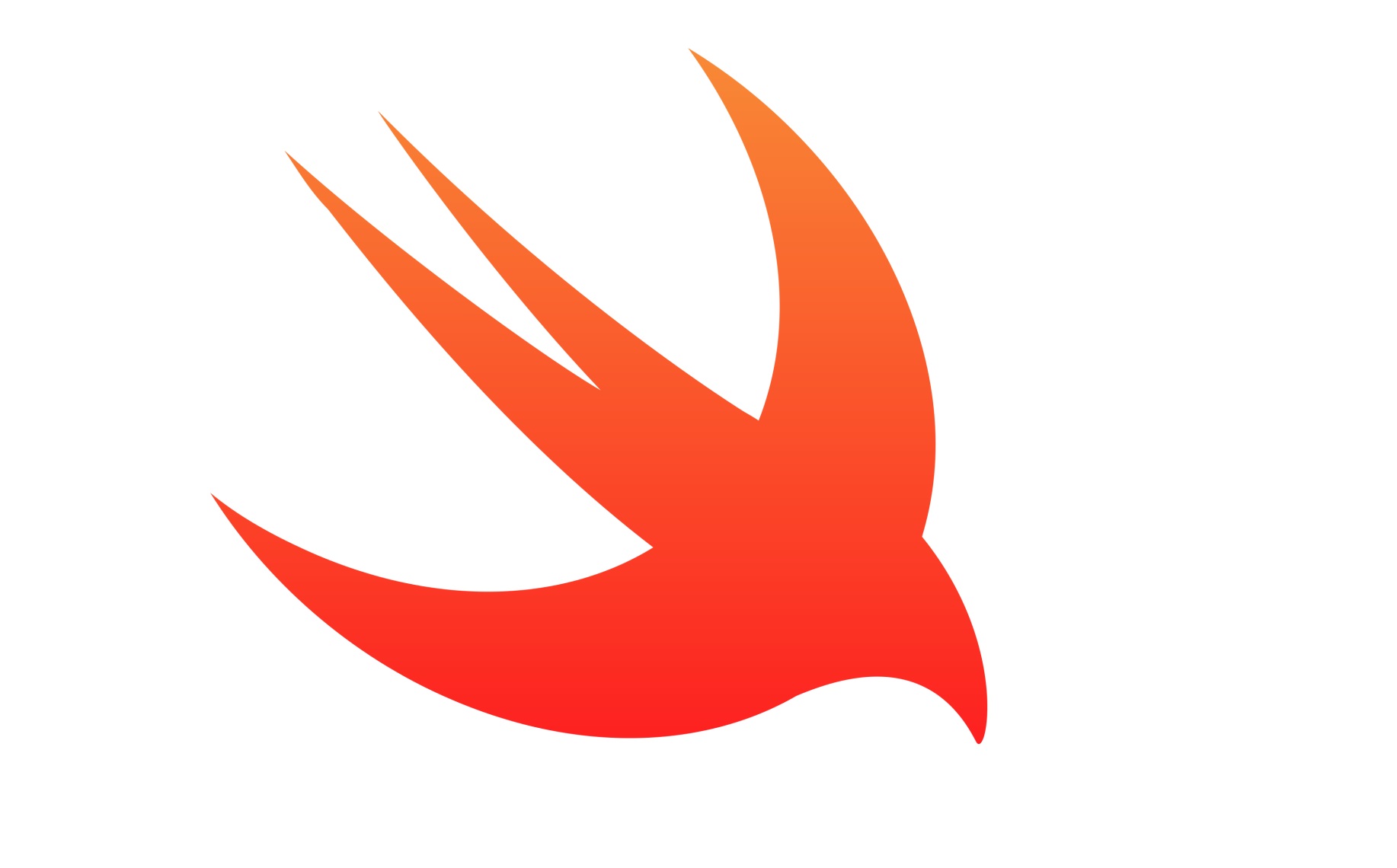
Swift programming language is a programming language developed by Apple and released in 2014. It is designed to provide a modern, secure and powerful alternative to Objective-C, which was previously primarily used for developing apps for iOS and macOS platforms has been used.
Swift was designed to increase developer productivity while making code more understandable and less error-prone. The language combines concepts from various programming languages and has been optimized by Apple for developing applications on its platforms.
characteristic | Explanation |
---|---|
developer | Developed by Apple |
publication | 2014 |
Purpose | Modern, secure and powerful programming language for iOS and macOS platforms |
alternative to | Objective-C, previously used primarily for developing apps on Apple platforms |
Security | Uses modern approaches to error prevention and handling, optionals, type inference and strong typing |
simplicity | Clear and shorter syntax compared to Objective-C, makes reading and writing code easier |
Performance | Optimized for high performance on Apple platforms, leveraging modern compiler optimizations for fast and efficient code |
Interoperability | Seamlessly integrates with existing Objective-C code, enabling gradual migration to Swift without a complete rewrite |
Applications | Use for iOS, macOS and server application development |
Support | Active developer community, regular updates and new features from Apple |
Some features of Swift are:
- Security: Swift uses modern approaches to error prevention and handling to ensure the code is as robust as possible. It includes features such as optionals, type inference and strong typing.
- Simplicity: Swift is designed to provide clearer and concise syntax compared to Objective-C. This makes the code easier to read and write.
- Performance: Swift is optimized to achieve high performance on Apple platforms. The language uses modern compiler optimizations to generate fast and efficient code.
- Interoperability: Swift can be used seamlessly with existing Objective-C code, allowing developers to gradually transition from Objective-C to Swift without having to completely rewrite their existing projects.
Swift has evolved rapidly since its inception and has become a popular choice for developing iOS, macOS, and even server applications. The language is supported by an active developer community and Apple regularly provides updates and new features.
If you want to acquire new skills or expand your existing skills, Skillshare is for you. Please click here to access Skillshare's learning platform and gain new insights into a wide variety of topics.
The history of SWIFT
Swift's history begins with the release of Objective-C in 1983, a programming language used for developing apps on Apple platforms. Over the years, Objective-C became increasingly popular and became the primary language for developing iOS and macOS applications.
In 2010, Apple, under the leadership of Chris Lattner, began developing a new programming language that would be more modern, secure and more powerful than Objective-C. The project was started internally at Apple under the name “Swift”.
On June 2, 2014, Swift was officially launched at Apple's Worldwide Developers Conference (WWDC). The announcement surprised the developer community as few details were known about the new language at the time. Swift was intended to be an alternative to Objective-C and simplify the development of apps for iOS and macOS platforms.
The release of Swift was greeted with great interest by the developer community. The new language promised many improvements, such as clearer and shorter syntax, improved error handling, better performance and higher security compared to Objective-C.
Apple made Swift open source, meaning developers had access to the source code, could contribute improvements, and extend the language on other platforms. This led to a rapidly growing community of developers supporting Swift and helping to further develop the language.
Since Swift was released, Apple has regularly released updates and new features for the language. Swift quickly became a popular choice for iOS, macOS, and server application development. Many developers have successfully migrated existing Objective-C code to Swift or started developing their projects from scratch using Swift.
Swift's history is one of continuous development and innovation. The language has helped transform the way developers build apps for Apple platforms and has created a vibrant and active community of developers committed to Swift.
Destination of SWIFT
Swift aims to provide a modern, secure and powerful programming language for developing apps on Apple platforms (such as iOS, macOS, watchOS and tvOS). Swift was designed to increase developer productivity while making code more understandable and less error-prone. Here are some of Swift's main goals:
- Modernity: Swift should withstand modern programming languages and support the latest language features and paradigms. By integrating modern concepts and syntax elements, Swift is able to offer developers a contemporary programming experience.
- Security: An important goal of Swift is the security of the code. The language has features such as optionals, type inference, and strong typing that help developers avoid potential errors. Swift consciously promotes the secure handling of storage and data, resulting in more robust and stable applications.
- Simplicity: Swift is designed to provide clearer and concise syntax compared to Objective-C. This makes reading and writing code easier and helps improve developer productivity. By reducing complexity, developers can work more efficiently and focus on solving problems.
- Performance: Swift is optimized for high performance on Apple platforms. The language uses modern compiler optimizations to generate fast and efficient code. This allows developers to create powerful and responsive applications that meet Apple's high standards.
- Interoperability: Swift is designed to work seamlessly with existing Objective-C code. This allows developers to gradually transition from Objective-C to Swift and leverage existing resources and libraries without having to completely rewrite their existing projects.
By achieving these goals, Swift aims to make developing apps on Apple platforms more effective, safer and faster. The language has helped make app development more accessible and engaging for millions of developers worldwide.
Syntax von SWIFT
Language construct | Example | Explanation |
---|---|---|
variables | var myVariable = 42 | Declaration of a mutable variable with an initial value |
Constants | let myConstant = "Hello, World!" | Declaration of an immutable constant with an initial value |
Features | ```swift | Declaration of a function with optional parameters and a return value |
func greet(name: String = "World") -> String { | ||
return "Hello, (name)!" | ||
} | ||
let greeting = greet(name: "John") | Function call with a specific argument | |
Conditions | ```swift | Conditional statements with if , else if and else |
let age = 25 | ||
if age < 18 { | ||
print("You are a minor.") | ||
} else if age >= 18 && age < 65 { | ||
print("You are an adult.") | ||
} else { | ||
print("You are a senior.") | ||
} | ||
Grind | ```swift | Grind with for-in and while |
for i in 1...5 { | ||
print(i) | ||
} | ||
var counter = 0 | ||
while counter < 10 { | ||
print(counter) | ||
counter += 1 | ||
} | ||
Optionals | ```swift | Declaration of an Optional value that is either a value or nil may have |
var optionalName: String? = "John" | ||
if let name = optionalName { | Unwrapping the optional with a conditional assignment | |
print("Hello, (name)!") | ||
} else { | ||
print("Name is nil.") | ||
} |
Swift Syntax is designed to provide a clear, readable and expressive programming language. Here are some important aspects of Swift syntax:
Type inference
Swift uses type inference to automatically determine the data type of variables and constants. This often allows you to omit the data type if it can be inferred from the assigned value. For example:
var number = 10 // The data type of 'number' is automatically inferred to 'Int'.
Variables and constants
Variables are declared using the var keyword and can change their value. Constants are declared with the let keyword and have a unique value that cannot be changed. For example:
var x = 5 let y = 10
Features
Functions in Swift are defined using the func keyword. They can contain parameters and optionally have a return value. The function definition is surrounded by curly brackets. For example:
func sayHello(name: String) { print("Hallo, (name)!") } sayHello(name: "John")
Conditional statements
Swift provides the if, else if and else statements for conditional executions. For example:
let age = 25 if age < 18 { print("You are a minor.") } else if age >= 18 && age < 65 { print("You are an adult.") } else { print("You are of retirement age.") }
Grind
Swift supports different types of loops, including the for-in loop and the while loop. For example:
for i in 1...5 { print(i) } var counter = 0 while counter < 10 { print(counter) counter += 1 }
Optionals
Optionals in Swift make it possible to deal with values that can potentially be nil. Optionally, adding a question mark ? displayed according to the data type. For example:
var optionalName: String? = "John"
Structures and classes
For example, classes and structures can be created.
// Struktur struct Person { var name: String var age: Int func sayHello() { print("Hello, my name is (name) and I am (age) years old.") } } let person = Person(name: "Max", age: 25) person.sayHello() // Class class Vehicle { was brand: String init(brand: String) { self.brand = brand } func honk() { print("Hooonk!") } } let car = Vehicle(brand: "BMW") car.honk()
Advanced concepts in SWIFT
- Generics
- Protocols
- Extensions
- Optionals
SWIFT in practice
Development environments
Various development environments (IDEs) and code editors are available for developing with Swift. Here are some of the most popular options:
- Xcode: Xcode is Apple's official development environment and provides comprehensive support for developing Swift applications for iOS, macOS, watchOS and tvOS. It includes a code editor, interface builder, debugging tools, simulators and much more.
- AppCode: AppCode is an IDE developed by JetBrains specifically designed for Swift, Objective-C and C/C++ development. It offers rich functionality such as code completion, refactoring tools, debugging support, and integration with other tools.
- Visual Studio Code: Visual Studio Code is a cross-platform code editor from Microsoft that can also be used for Swift development. Extensions such as Swift Language or SourceKit-LSP can be used to add Swift support, including syntax highlighting, code completion, and debugging.
- Atom: Atom is an open source code editor from GitHub that can be customized through extensions to meet the needs of Swift development. Swift packages such as "language-swift" or "ide-swift" are available that provide support for syntax highlighting and other features.
- Sublime Text: Sublime Text is a popular cross-platform code editor that can be customized for Swift development through extensions. There are packages like "Swift" or "SwiftLanguageSupport" that provide features like syntax highlighting, code completion, and error detection.
There are other options such as CLion, Vim with Swift extensions or Eclipse with the Swift Development Tools plugin. The choice of development environment depends on your individual preferences, the operating system and the specific requirements of your project.
Frameworks and libraries
There are a variety of frameworks and libraries that can be used in Swift development to facilitate application development and add additional functionality. Here are some of the most common frameworks and libraries for Swift:
- UIKit: The UIKit framework is the primary framework for building user interfaces for iOS, iPadOS, and macOS applications. It provides a variety of classes and functions to create and manage user interface components such as views, buttons, labels, tables and much more.
- SwiftUI: SwiftUI is a modern framework for declarative user interface development in Swift. It allows creating user interfaces with a simple and intuitive code. SwiftUI supports both iOS and macOS and offers a variety of user-friendly features and approaches.
- Alamofire: Alamofire is a popular HTTP networking library for Swift. It provides a simplified API for executing network requests, processing JSON data, and uploading files. Alamofire makes communicating with web APIs easier and more efficient.
- CoreData: CoreData is a framework for managing databases and storing data in Swift applications. It provides a powerful API for creating, querying and updating database objects and allows working with persistent data storage.
- SpriteKit: SpriteKit is a framework for creating 2D games and interactive graphics applications in Swift. It offers animation, physics simulation, collision detection and more features. SpriteKit allows developers to create fun and engaging games.
- CoreML: CoreML is a framework that machine learning (ML) in Swift applications. It facilitates the integration of trained ML models for tasks such as image recognition, speech recognition or text analysis. CoreML allows developers to incorporate ML capabilities into their applications.
- SwiftyJSON: SwiftyJSON is a library that makes working with JSON data in Swift easy. It provides a simple API for parsing and processing JSON objects, making it easier to work with JSON data.
These are just a few examples of frameworks and libraries that can be used in Swift development. There are many other options that cover more specific functionality, such as Realm for databases, AlamofireImage for loading images from the network, Firebase for backend integration, and many more. The choice of frameworks and libraries depends on the requirements of your project and your individual preferences.
Best Practices
There are a number of tried and tested methods when developing with Swift
, which can help you write high-quality and maintainable code. Here are some important Swift best practices:
- Consistent naming: Use meaningful and consistent names for variables, functions, classes, and other code elements. Follow Swift naming conventions and use clear and understandable names to make the code easier to read and understand.
- Avoid overly long features: Try to keep features short and focused on a specific purpose. Long functions can be difficult to read and understand. A good practice is to break functions into smaller, reusable parts and group blocks of code with specific tasks.
- Use type inference where it makes sense: Swift provides type inference to automatically determine the data type of variables and constants. Use this feature to make the code cleaner and more readable. However, use explicit types if it improves code clarity or security.
- Use Optionals and Safe Unwrapping: Optionals help deal with values that may
nil
could be. Use optional and safe unwrapping to ensure you don't have any untreatednil
-cases and make the code more robust. - Use enums instead of magic values: Use enums to avoid magic values and make code more readable. Define enums for concrete values or states to reduce errors and improve code maintainability.
- Use Swift Options for Objective-C code: If you use Objective-C frameworks or libraries in Swift, use Swift Options to ensure you maintain Optionals and Type-Safety in your Swift codebase.
- Write detailed and understandable comment code: Comments help document the code and explain to other developers what the code does and why it is implemented in a certain way. Write clear, concise, and understandable comments to make code more readable and improve maintainability.
- Regular code reviews and testing: Conduct regular code reviews to identify potential bugs, style issues, and other opportunities for improvement. Also perform extensive testing to ensure that the code works correctly and meets expected requirements.
These best practices are designed to help you write high-quality, maintainable Swift code. It's important that you also consult the official Swift documentation, developer communities, and testimonials from other developers to continually learn from best practices and new insights.
Debugging und Testing
Debugging and testing in Swift is done using various tools and frameworks to find errors, review the code, and ensure that it works correctly. Here are the common approaches to debugging and testing in Swift:
Debugging:
- Xcode Debugger: Xcode, the official development environment for Swift, provides a powerful debugger. You can set breakpoints, monitor program flow, view variable values, and navigate through the code step by step to find errors and analyze the code.
- Console Outputs: By adding output messages with
print()
-Directions placed strategically in the code allow you to display information in the Xcode console during execution. This will help you monitor the health of the program and identify potential sources of errors.
Testing:
Unit Tests: Swift supports writing unit tests using the XCTest framework built into Xcode. You can create test cases, test functions and methods, and use assertions to ensure that code works as expected. Unit tests help to detect errors early and validate the functionality of individual code sections.
- UI Testing: The XCUITest framework allows you to write UI tests for iOS apps. You can simulate the user interface, perform user interactions, and check the app's reactions. This allows you to check the correct functioning and behavior of the app from the user's perspective.
- Mocking and Stubbing: Swift offers various frameworks such as XCTest, Quick and Nimble that enable mocking and stubbing. These tools allow you to simulate or modify dependencies to test specific scenarios and ensure isolation of code units.
It is important to integrate both debugging and testing into your development practice to detect errors early and ensure code stability and quality. Through careful testing and thorough debugging, you can improve the reliability of your Swift applications.
Future of SWIFT
Swift's future looks bright as the language has strong support from Apple and an active developer community. Here are some aspects that point to Swift's future:
- Evolution and Improvements: Apple is committed to continually developing and improving Swift. New versions and updates are released regularly, bringing new features, performance improvements, stability and security improvements, and bug fixes.
- Cross-platform support: Swift is no longer just used for iOS and macOS development. There are efforts to make Swift available for other platforms such as Linux and Android. This opens up new opportunities for developers to deploy Swift-based solutions on a wider range of devices and operating systems.
- Open source community: Swift is an open source programming language, meaning developers worldwide can contribute to the source code and extend the language to other platforms. The active and growing developer community helps further develop the language, provide additional libraries and tools, and strengthen the ecosystem around Swift.
- Use in new areas: Swift is not only used for developing iOS and macOS apps. It is also increasingly being used for server-side applications, machine learning, data science and other areas. As Swift continues to evolve and optimize, the language will become better suited to meet the needs and requirements in these areas.
- Continued strong support from Apple: Because Swift was developed by Apple, it will continue to have tight integration with Apple platforms. Apple continues to bet heavily on Swift and promotes its use for developing apps and services on their devices. This ensures that Swift will play an important role in Apple's developer landscape.
Overall, the combination of Apple's support, Swift's open source nature, and growing developer community suggests that Swift will continue to be a popular and powerful programming language in the future.