JavaScript: The all-round programming language for web development and more
Published
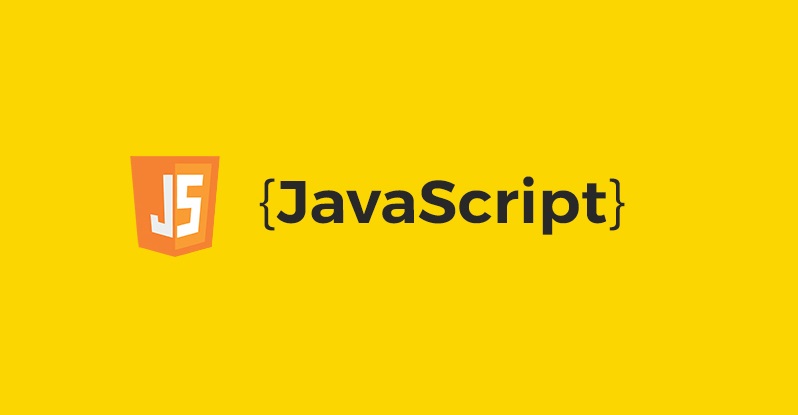
JavaScript is a widely used scripting language primarily used for developing web applications. It is an interpreted, object-oriented language that is primarily used for client-side development of interactive websites.
If you want to refresh your JavaScript knowledge or even further expand it, we recommend you Skillshare as a learning platform.
JavaScript allows developers to create interactive elements on the website and control user interactions. This includes functions such as modifying HTML content, responding to user actions (such as mouse clicks or keyboard input), validating forms, animating elements, and communicating with the server via asynchronous requests (AJAX).
Over the years, JavaScript has evolved and is now also used for server-side development (with platforms such as Node.js), as well as mobile and desktop application development (with frameworks such as React Native and Electron).
JavaScript is an interpreted language that runs directly in the web browser. Every modern web browser contains a JavaScript interpreter that interprets and executes the code at runtime. This allows developers to directly access client-side code and improve user experience without the need for additional plugins or installations.
JavaScript has a large and active developer community and an extensive collection of libraries and frameworks that make development easier. Popular JavaScript frameworks include React, Angular and Vue.js. These frameworks provide advanced features for developing web applications, including handling state management, building components, and implementing single-page applications.
Overall, JavaScript has a significant role in web development and in building interactive and dynamic websites and applications. It is a versatile language that is valued by many developers due to its widespread support and wide range of applications.
Why is JavaScript important?
JavaScript is important for several reasons:
- Client-side interactivity: JavaScript allows developers to make web pages interactive and dynamic. It allows adding effects, animations, form validation and other interactive features that improve user experience. Without JavaScript, websites would be static and unable to handle user interactions.
- Web Applications: JavaScript is the primary programming language for developing web applications. By using JavaScript, developers can create complex applications that run on the client (in the web browser). JavaScript can be used to develop modern web applications such as email clients, social media platforms, online shops and collaboration tools.
- Cross-platform development: JavaScript enables the development of applications that can run on different platforms. Frameworks like React Native and Electron allow developers to build mobile apps for iOS and Android, as well as desktop apps for Windows, macOS, and Linux using JavaScript. This enables code reuse and simplifies development for multiple platforms.
- Extending websites and frameworks: JavaScript can be used to extend existing websites and frameworks. By incorporating JavaScript libraries and frameworks, developers can integrate additional functions and features into their websites. This makes it easy to integrate maps, charts, calendars, chat functions and much more.
- Real-time updating: With JavaScript and the corresponding technologies such as WebSockets or server-sent events, websites can be updated in real time. This allows changes and updates to be viewed immediately without the need to reload the page. Real-time updates are especially important for applications such as real-time chats, collaboration tools, and data feed updates.
- Supported by a large community and extensive resources: JavaScript has an enormous and active developer community. This means there is a wealth of resources, documentation, tutorials, forums and libraries to help developers expand their JavaScript skills and overcome challenges. The large community also promotes innovation and regular development of the language and its tools.
JavaScript has become an essential component of web development due to its wide range of applications, wide support, and interactivity it enables in web applications. It plays a key role in designing user experiences, developing web applications, and expanding the possibilities of the web.
How is JavaScript used?
JavaScript is used in different ways:
- Client-side web development: JavaScript is primarily used to extend the functionality of web pages on the client side. Developers use JavaScript to control user interactions, HTML -Dynamically change content, validate forms, add animations and respond to events such as mouse clicks and keystrokes. JavaScript makes it possible to make websites interactive and responsive.
- Web Application Development: JavaScript is the primary language for developing web applications. Using JavaScript frameworks and libraries such as React, Angular, and Vue.js, developers can build modern single-page applications (SPA) that provide a seamless user experience. JavaScript allows communicating with APIs, managing state, and rendering components on the client.
- Server-side development: With the introduction of Node.js, JavaScript has also found its way into server-side development. Node.js allows developers to build server-side applications and APIs using JavaScript. This allows both client-side and server-side logic to be written in the same language and code to be shared between client and server.
- Mobile Applications: Using React Native, a JavaScript framework, developers can develop mobile applications for iOS and Android. React Native makes it possible to write most of the code in JavaScript and then convert it into native UI components for the respective platforms. This makes it easier to develop cross-platform mobile applications.
- Desktop Applications: Electron allows developers to build desktop applications for Windows, macOS and Linux using JavaScript, HTML and create CSS. Electron leverages the same technologies used for web development to create cross-platform desktop applications.
- Data visualization and manipulation: JavaScript is widely used for data manipulation and visualization in web applications. Libraries like D3.js, Chart.js, and Plotly enable developers to represent and process data in charts, graphs, and interactive visualizations.
It is important to note that JavaScript is used in a variety of fields and technologies and is constantly evolving. The use of JavaScript is not limited to the above applications but may vary depending on the needs and goals of a project.
Basics
The basics of JavaScript include the following concepts and elements:
Data structure | Description |
---|---|
Array | An ordered collection of elements accessible through indexes. |
Object | A collection of key-value pairs used to organize and store data. |
Set | A collection of unique values that contains no duplicates. |
Map | A collection of key-value pairs that allows access to values based on a unique key. |
WeakSet | A special type of set that contains only weak references to its elements. |
WeakMap | A special type of map that contains only weak references to its key-value pairs. |
Stack | A data structure that operates on a last-in-first-out (LIFO) basis, where the last element added is the first to be removed. |
Queue | A data structure that operates on a first-in-first-out (FIFO) basis, where the element added first is removed first. |
Linked List | A data structure where each element (node) has a connection to the next element. |
Binary Tree | A tree with at most two children per node. |
Graph | A collection of nodes connected by edges. |
Hash Table | A data structure that stores key-value pairs and enables fast access and insert operations. |
Trie | A tree-like data structure used to store and search strings efficiently. |
Heap | A data structure organized as a complete binary tree that satisfies certain heap properties. |
Doubly Linked List | A variant of the linked list in which each element has a connection to the previous and next element. |
- Variables: JavaScript uses variables to store values. Variables are declared by the keyword "var", "let" or "const" and can contain various data types including numbers, strings, booleans, arrays, objects and more.
- Data Types: JavaScript supports various data types such as numbers (e.g. integers and floating point numbers), strings (text), Booleans (true/false), arrays (lists of values), objects (summary data), and special values such as "undefined" and "null ".
- Operators: JavaScript provides various operators to perform mathematical calculations, perform comparisons, and perform logical operations. Examples of operators are "+", "-", "*", "/", ">", "<", "===" and "&&".
- Control Structures: JavaScript supports control structures such as conditions (if-else statements), loops (for loops, while loops), and switch statements. These allow the program flow to be controlled based on certain conditions.
- Functions: Functions in JavaScript make it possible to define a block of code that can be reused. Functions can accept parameters, return values and can also be defined anonymously (without a name). Functions are fundamental to building modules and organizing code in JavaScript.
- Objects: Objects can be created in JavaScript to organize data and functionality. Objects consist of properties (key-value pairs) and methods (functions). Objects can also be inherited to create inheritance hierarchies.
- Event Handling: JavaScript allows you to respond to events that occur related to user interactions, such as mouse clicks, keystrokes, and webpage loading. Event handling allows actions to be executed when a specific event occurs.
- DOM Manipulation: The Document Object Model (DOM) allows the manipulation of HTML elements and CSS styles using JavaScript. Accessing the DOM allows elements to be selected, modified, and dynamically created or removed.
These are just a few basic concepts and elements of JavaScript. There are many other functions, libraries and frameworks that facilitate and extend development in JavaScript. It's important to understand the basics of JavaScript to build solid programming skills and use the language effectively.
DOM Manipulation
DOM manipulation refers to changing, adding or removing HTML elements and CSS styles using JavaScript by accessing the Document Object Model (DOM). The DOM is a programming interface that enables a structured representation of an HTML document. It represents the HTML elements as a tree structure, where each element is a node in the tree.
DOM manipulation is important for creating dynamic websites and web applications. JavaScript can be used to access the DOM to select elements, change their properties or contents, create new elements, or remove existing elements. This allows developers to control user interactions, dynamically update content, and improve user experience.
Here are some examples of DOM manipulation with JavaScript:
- Select elements: JavaScript allows you to select specific HTML elements by using their IDs, classes, tags, or other selectors. For example:
javascript Copy code
var element = document.getElementById("myElement"); var elements = document.getElementsByClassName("myClass"); var element = document.querySelector("div"); var elements = document.querySelectorAll(".myClass");
- Change content: JavaScript allows you to change the content of HTML elements. For example:
javascript Copy code
var element = document.getElementById("myElement"); element.innerHTML = "Neuer Inhalt";
- Change styles: JavaScript allows you to change the CSS styles of HTML elements. For example:
javascript Copy code
var element = document.getElementById("myElement"); element.style.color = "red"; element.style.fontSize = "20px";
- Creating and adding elements: JavaScript allows you to create new HTML elements and add them to the DOM. For example:
javascript Copy code
var newElement = document.createElement("div"); newElement.innerHTML = "New Element"; document.body.appendChild(newElement);
- Remove elements: JavaScript allows you to remove existing HTML elements from the DOM. For example:
javascript Copy code
var element = document.getElementById("myElement"); element.parentNode.removeChild(element);
DOM manipulation is a powerful tool to dynamically change the structure, content and appearance of web pages. It allows interaction with the user and updating the webpage based on various events and conditions.
AJAX
AJAX stands for "Asynchronous JavaScript and XML" and is a technique for exchanging data between the browser and the server without having to reload the entire website. It enables asynchronous communication between the client (web browser) and the server, resulting in an improved user experience.
AJAX allows web applications to retrieve data from the server without reloading the current page. This allows dynamic reloading of content, refreshing parts of the page, and performing actions in the background without interrupting the user. Instead of receiving a full HTML page, the server typically sends back data in JSON or XML format, which is then included JavaScript can be processed in the browser.
Using AJAX makes it possible to make web pages faster and more responsive by only loading the data needed instead of re-rendering the entire page. This results in a better user experience as pages load faster and user interactions can take place without visible interruptions.
JavaScript frameworks and libraries such as jQuery, Axios and Fetch API provide functions and methods to simplify AJAX communication. They abstract the complexity of AJAX requests and enable easy handling of asynchronous requests in code.
It is important to note that AJAX is not limited to XML, although it appears in its name. XML was widely used as a data format for communication in the past, but today JSON (JavaScript Object Notation) or other data formats are more commonly used.
AJAX has revolutionized the way web applications are developed and contributes to the development of interactive and dynamic web pages by enabling seamless communication between the client and the server without the need to reload the page.
How is AJAX used?
AJAX (Asynchronous JavaScript and XML) is used to exchange data asynchronously between the web browser (client) and the server without having to reload the entire web page. Here is a basic flow of how to use AJAX:
- Creating an XMLHttpRequest instance: First, an XMLHttpRequest instance is created that enables communication with the server. Modern JavaScript libraries and frameworks like Fetch API simplify this step and provide a more user-friendly API.
- Setting a callback function: A callback function is defined that will be called when the AJAX request completes and the data is returned from the server.
- Configuring the AJAX request: The AJAX request is configured to specify the server endpoint, the type of request (GET, POST, PUT, DELETE, etc.), any parameters and other request options.
- Sending the AJAX request: The AJAX request is sent with the
send()
method sent. Data can be sent to the server if necessary. - Processing the server response: Once the server response is received, the defined callback function is called. In this function, the received data can be processed and inserted into the web page to update it dynamically.
The typical AJAX flow includes the following steps:
- Capturing user actions: The user interacts with the website, for example by clicking a button or filling out a form.
- Triggering AJAX request: Based on the user action, an AJAX request is generated and sent to the server.
- Processing the server response: Once the server processes the request, it sends back a response. The received response is processed by the callback function and the web page is dynamically updated to display the new data or updated content.
- Refresh the web page: The web page is updated based on the received data or the response from the server without having to reload the entire page.
AJAX is widely used in web applications to enable asynchronous data updates, live searches, real-time chat, and many other features that provide a seamless user experience. Modern JavaScript libraries and frameworks simplify the use of AJAX and provide powerful functions and methods for data transfer and processing.
What is meant by XMLHttpRequest?
XMLHttpRequest is an object built into JavaScript that makes it possible to send HTTP requests asynchronously and exchange data between the web browser (client) and the server. It is an essential part of AJAX (Asynchronous JavaScript and XML) and allows communication with a server without having to reload the entire web page.
The XMLHttpRequest object provides an interface for creating, configuring, and executing HTTP requests. Here are some important methods and properties of the XMLHttpRequest object:
-
open(method, url, async)
: This method is used to open a connection to a specific URL. The "method" parameter specifies the HTTP method, such as GET or POST. "url" is the address of the server to send the request to. "async" determines whether the request is sent asynchronously or synchronously. -
setRequestHeader(header, value)
: This method is used to add custom HTTP headers to the request, such as "Content-Type" or "Authorization". -
send(data)
: This method sends the request to the server. Optionally, data can be passed as a parameter, e.g. if a POST request with form data is to be sent. -
onreadystatechange
: This property allows setting a callback function that will be called when the status of the request changes. The callback function is usually used to process the server response. -
responseText
andresponseXML
: These properties contain the server response as a text or XML document, depending on the type of content received. -
status
andstatusText
: These properties return the HTTP status code and corresponding text description of the server response.
XMLHttpRequest can be used to retrieve data from the server, send data to the server, and respond to events such as request progress and request status change. It enables asynchronous communication between the client and the server, which helps in developing interactive and responsive web applications.
However, it is important to note that XMLHttpRequest is considered an older method of AJAX communication. Modern web applications more commonly use the Fetch API or JavaScript libraries such as Axios, which are built on top of XMLHttpRequest and provide a simpler and more flexible API.
What is meant by JSON?
JSON (JavaScript Object Notation) is a lightweight data format used to represent data in a structured manner. It is a common format for exchanging data between client and server in web applications.
Here is some important information about JSON:
- Syntax: JSON is based on a syntax similar to JavaScript object syntax. It consists of key-value pairs, where the key can be a string and the value can be any data type, including numbers, strings, booleans, arrays, objects, and special values such as null. JSON data is usually enclosed in curly brackets {} or square brackets [].
- Data representation: JSON provides an easy way to represent complex data structures. Objects can be nested by placing them within an object or array. This allows complex data structures to be created that can be easily serialized and transmitted.
- Readability: JSON is easily readable and understandable by humans. The syntax is simple and intuitive, making troubleshooting and debugging easier.
- Data transfer: JSON is often used to exchange data between client and server in web applications. It is platform independent and can be interpreted by various programming languages, not just JavaScript. Most modern programming languages provide functions for encoding (serializing) and decoding (deserializing) JSON data.
- Data processing: JSON is a flexible format that offers a variety of data processing options. It can be easily converted into JavaScript objects and manipulated. JSON is often used to create APIs and retrieve and display data from external sources such as web services.
- Support: JSON is supported by most modern programming languages and frameworks. There are built-in functions and libraries that make encoding and decoding JSON data easier. In JavaScript the global one is
JSON
object reference that provides methods for parsing and creating JSON data.
JSON provides a simple and effective way to structure and transmit data. It is easily readable by both humans and machines and has established itself as a common data format in web development.
Frameworks and libraries
Framework | Description |
---|---|
React | A powerful UI framework for user interface development. |
Angular | A comprehensive framework for developing web applications. |
View.js | A lightweight framework for developing interactive user interfaces. |
Ember.js | A framework for ambitious web applications with a focus on conventions. |
Backbone.js | A lightweight framework for structuring web applications. |
Express.js | A server-side framework for developing web applications and APIs based on Node.js. |
Meteor | A full-stack framework for developing web applications with integrated database access. |
Aurelia | A modern framework for developing web applications with a modular architecture. |
Polymer | A framework for developing web components and interactive user interfaces. |
D3.js | A powerful framework for data visualization on the web. |
Three.js | A framework for creating 3D graphics and interactive 3D applications on the web. |
Knockout.js | A framework for creating reactive user interfaces and data binding. |
Svelte | A Compiler based Framework for creating high-performance web applications. |
Next.js | A framework for developing server-side rendered React applications. |
Nuxt.js | A framework for developing server-side rendered Vue.js applications. |
Gatsby | A framework for developing fast, static websites and progressive web apps. |
There are a variety of JavaScript frameworks developed for different application areas and tasks. Here are some of the most famous and commonly used JavaScript frameworks:
- React: React, developed by Facebook, is a powerful user interface development framework. It enables the creation of interactive and reusable UI components and is used in a variety of web applications. React uses a virtual DOM structure to enable efficient and fast UI updates.
- Angular: Angular, developed by Google, is a rich JavaScript framework for developing web applications. It provides a complete infrastructure for building single-page applications (SPAs) and supports features such as data binding, dependency injection, routing and much more.
- Vue.js: Vue.js is a lightweight JavaScript framework for user interface development. It is used for creating interactive web applications and is characterized by simple syntax and low initial effort. Vue.js offers similar functionality to React and Angular, but in a more accessible way.
- Ember.js: Ember.js is a JavaScript framework for developing ambitious web applications. It offers a robust architecture and a rich collection of tools and features to build complex applications. Ember.js emphasizes convention over configuration and supports features such as data binding, routing, components, and more.
- Backbone.js: Backbone.js is a lightweight JavaScript framework used to structure web applications. It provides a simple way to organize code and supports models, views and events. Backbone.js integrates well with other libraries and frameworks and enables the creation of simple to medium-sized web applications.
- Express.js: Express.js is a server-side JavaScript framework for developing web applications and APIs. It is based on Node.js and provides a simple and flexible way to create web servers and define routes. Express.js is often used as a core component in developing Node.js applications.
These are just a few of the most popular JavaScript frameworks, but there are many more that cover specific use cases and needs. Choosing the right framework depends on the needs of the project, development preference, and stage of development. It's important to consider each framework's pros and cons and review their documentation and community support to make the best choice.
Debugging und Testing
There are various debugging tools and techniques specifically designed for troubleshooting and debugging JavaScript applications. Here are some of the most popular debugging tools for JavaScript:
- Browser developer tools: Modern web browsers such as Google Chrome, Mozilla Firefox, Microsoft Edge and Safari have built-in developer tools. These tools provide extensive functionality for debugging JavaScript code, including checking code errors, monitoring network requests, inspecting DOM elements and styles, stepping through code, setting breakpoints, and much more.
- Console: The JavaScript console is a simple but powerful debugging tool included in the browser's developer tools. Developers can use the console to enter and execute JavaScript code, review error messages and output, monitor variable values, and test functions. The console is a valuable tool for quickly testing code and viewing debugging information.
- Debugger statement: JavaScript provides the
debugger
statement that can be inserted into the code to set a breakpoint. When the code encounters this instruction, execution will stop and the browser's developer tools will automatically open. This allows checking variable values, step-by-step code execution, and investigating errors. - Linting tools: Linting tools like ESLint help identify potential errors, style issues, and best practices in JavaScript code. They check the code for errors and issue warnings or error messages. These tools help find errors in code before it runs and promote consistent code quality.
- Remote debugging: When developing JavaScript for mobile devices or other platforms, remote debugging can be very useful. It allows developers to debug the code on a remote device or environment while accessing their local developer tools. This makes troubleshooting in specific environments easier and allows problems to be checked on different platforms.
- Profiling tools: Profiling tools help analyze the performance of JavaScript applications and identify bottlenecks or inefficient code. They provide insights into resource consumption, function execution time, and other performance metrics. Examples of profiling tools include Chrome DevTools Profiler and Firefox Performance Monitor.
These debugging tools provide developers with the ability to effectively debug and find errors in JavaScript applications. It is advisable to use different tools to get the best debugging experience and improve development efficiency.