Java vs Python
Published
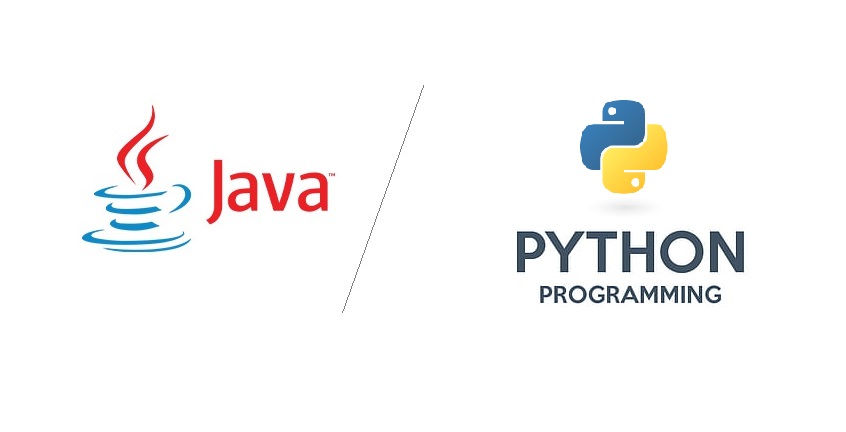
Java and Python are two popular programming languages that differ in several aspects. Here are some of the main differences between Java and Python:
Aspect | Python | Java |
---|---|---|
Typing | Dynamically typed: Variables are typed at runtime | Statically typed: Variables must specify a type when declared |
Syntax | Simple and easy to read syntax with fewer lines of code | Uses a stricter syntax with a larger number of lines of code |
Speed | Usually slower than Java due to runtime interpretation | Usually faster than Python due to direct compilation to machine code |
Areas of use | Scripting, data analysis, web development, artificial intelligence (AI), machine learning | Enterprise applications, server development, mobile applications, Internet of Things (IoT) |
Community and libraries | Large and active community with extensive libraries (e.g. NumPy, Pandas, Django) | Large and established community with extensive libraries (e.g. Spring, Hibernate) |
Simplicity | Simple syntax and less boilerplate code | Rather more complex syntax and more required code |
Platform independence | Python is platform independent and can run on different operating systems | Java is platform-independent and can run on different operating systems |
Learning curve | Python has a flatter learning curve and is often easier for beginners to learn | Java has a steeper learning curve and can be more complex for beginners |
- Typing: Java is statically typed, which means that variables must specify a certain type when declared and this cannot be changed during runtime. Python, on the other hand, is dynamically typed, which means that variables can be assigned a type at runtime and type changes are permitted.
- Syntax: Python features a simpler and easy-to-read syntax that requires fewer lines of code. Java, on the other hand, uses a stricter syntax with a larger number of lines of code.
- Speed: Java is generally faster than Python because Java code is compiled directly into machine code. Python, on the other hand, is interpreted at runtime, which can result in slower execution speed.
- Areas of use: Python is often used in areas such as scripting, data analysis, web development, artificial intelligence (AI), and machine learning. Java, on the other hand, is often used for enterprise applications, server development, mobile applications, and the Internet of Things (IoT).
- Community and libraries: Both languages have large and established developer communities. However, Python has a particularly active community and has extensive libraries that cover specific task areas, such as NumPy and Pandas for data analysis or Django for web development. Java also has a wide range of libraries, including Spring and Hibernate for enterprise application development.
- Simplicity: Python is often considered an easy language to learn with a flatter learning curve, making it more accessible to beginners. Java, on the other hand, has a steeper learning curve and can be more complex for beginners due to concepts such as classes, object orientation, and the use of development environments.
It is important to note that both Java and Python have their strengths and areas of use, and the choice of programming language depends on the specific requirements of the project, existing infrastructure, developer preference, and other factors.
If you want to refresh your Java/Python knowledge or even expand it further, we recommend Skillshare as a learning platform.
Performance
In terms of performance, there are some differences between Java and Python:
- Execution speed: In general, Java is faster than Python. Java code is compiled directly into machine code, while Python code is interpreted at runtime. The interpretation of Python code can result in some slowdown, especially for computationally intensive operations. Java, on the other hand, benefits from direct compilation and can therefore have a higher execution speed.
- Memory usage: Python typically uses more memory than Java. This is partly due to the dynamic typing and memory management in Python. Java, on the other hand, uses efficient memory management that optimizes memory usage and typically has lower memory usage.
- Parallel processing: Java provides better support for parallel processing than Python. Java has dedicated libraries and tools such as Threads, Executor Framework, and Fork/Join that allow tasks to be processed in parallel. Python also has parallelization capabilities, but the implementation is typically more complex and less seamless than Java.
It is important to note that the performance of a programming language depends on several factors, including the efficiency of the code written, the optimization of the algorithms, the hardware on which the code is run, and the specific requirements of the project. In many applications and scenarios, Python's performance may be sufficient. However, when performance is a critical factor, especially in compute-intensive or time-sensitive applications, Java may offer an advantage due to its faster execution speed and efficient memory usage.
Syntax
Aspect | Java | Python |
---|---|---|
Block structure | Use of curly braces | Use of indentations |
Semicolons | Required at the end of each instruction | Optional to separate instructions |
comments | // for single line comments | # for single line comments |
/* */ for multiline comments | ''' ''' or """ """ for multiline comments | |
Variable declaration | Explicit specification of the data type | Dynamic typing |
Classes and methods | Use of the keyword "class | Use of the keyword "def |
Use of the "public" keyword | No explicit access modifier identifier | |
Conditions | if-else statements | if-else statements |
switch statements | Not available | |
Grinding | for loops | for loops, while loops |
while loops | ||
Arrays | Arrays with fixed size | Variable size lists |
(e.g. [element1, element2, ...]) | ||
Exceptions | try-catch blocks | try-except blocks |
throw statement | raise statement |
The syntax of Java and Python differs in several aspects. Here are some of the main differences:
- Block structure: Java uses curly braces ({}) to mark blocks of code, while Python uses indentations. In Python, code blocks are marked by indentations with spaces or tabs. Uniform indentations are required in Python to define the block structure.
Java example:
public class Beispiel {
public static void main(String[] args) {
// Code block in Java with curly braces
if (condition) {
System.out.println("Hallo");
} else {
System.out.println("World");
}
}
}
Python example:
# Codeblock in Python mit Einrückungen
if condition:
print("Hallo")
else:
print("Welt")
- Semicolon: In Java, a semicolon (;) is placed at the end of each statement to terminate it. In Python, semicolons are optional to separate statements. Instead, Python usually simply writes one statement per line.
Java example:
int zahl = 10;
// Semicolon at the end of the statement
System.out.println("Die Zahl ist: " + zahl);
Python example:
zahl = 10 # Semikolon ist optional
print("Die Zahl ist:", zahl)
- Data type declaration: In Java, variables must be declared with a specific data type, whereas in Python the typing is dynamic and variables do not require an explicit type declaration.
Java example:
int zahl = 10;
// Declaration of an integer
(int) String text = "Hallo";
// Deklaration einer Zeichenkette (String)
Python example:
zahl = 10
# Dynamic typing
text = "Hallo"
# Variablen haben keinen expliziten Typ
These examples show only some of the basic differences in the syntax of Java and Python. There are other differences, such as the use of keywords, the handling of conditions and loops, the definition of functions and classes, the handling of exceptions, etc. It is important to familiarize yourself with the specific syntax rules of each programming language in order to write correct and functional code.
Purpose
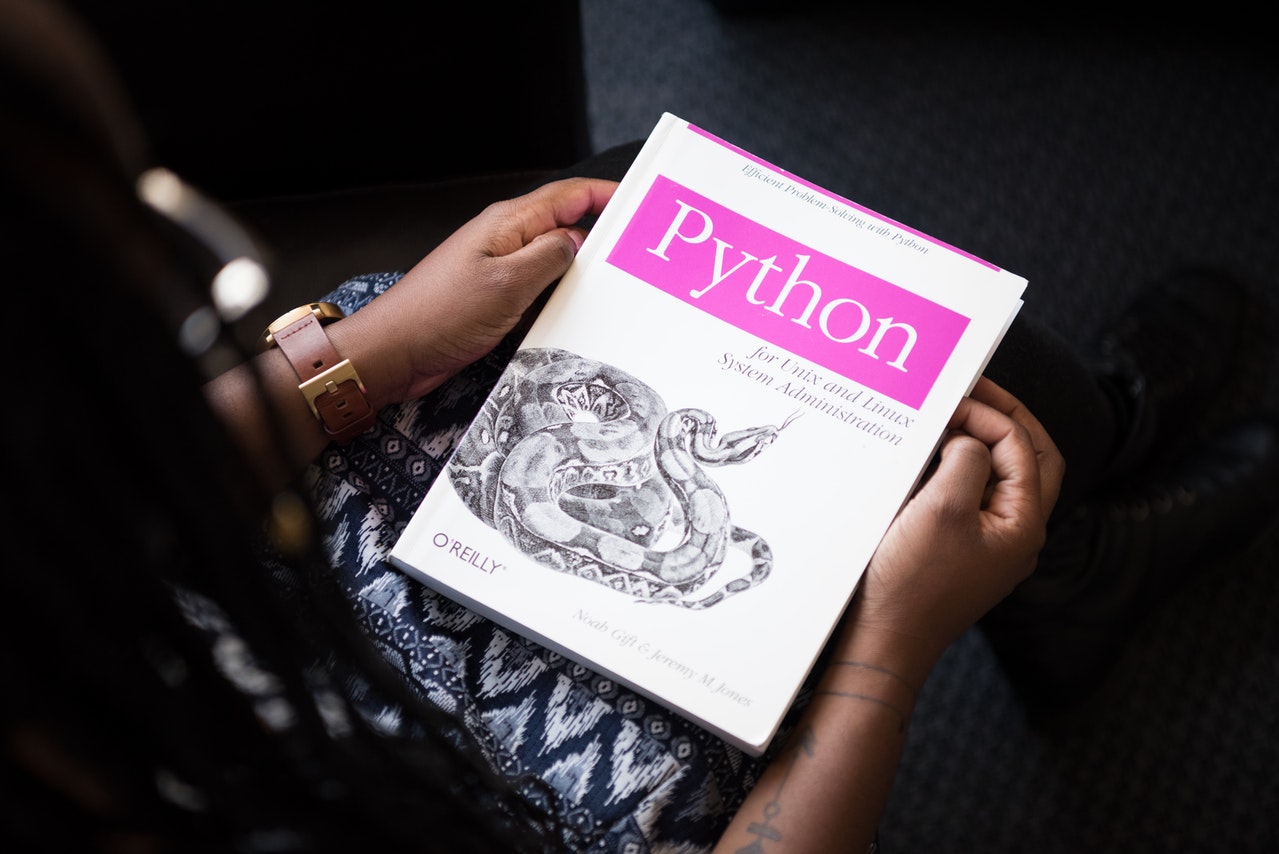
Quelle: pexels.com
Java and Python have different uses and are suitable for different application areas. Here are some of the common uses for each language:
Java:
- Enterprise applications: Java is widely used for enterprise application development, including large, complex systems, enterprise resource planning (ERP), customer relationship management (CRM), and more.
- Server-side development: Java is a popular choice for developing server-side applications, including web applications, application servers, and database servers.
- Android Development: Java is the primary programming language for Android application development.
- Financial services: Because of its stability and scalability, Java is widely used in the financial industry for applications such as trading platforms, banking systems, and payment processing.
- Internet of Things (IoT): Java finds application in the IoT development , especially in the programming of IoT devices and gateways.
Python:
- Data analysis and scientific calculations: Python is a leading language for data analysis, machine learning and artificial intelligence. Popular libraries such as NumPy, Pandas, scikit-learn and TensorFlow facilitate data analysis and modeling.
- Web Development: Python is used for web application development and frameworks like Django and Flask. It provides a simple and more readable syntax for web development.
- Automation and scripting: Python is ideal for automating repetitive tasks and writing scripts. It is widely used for system administration, data processing, and scripting tasks.
- Prototyping: Python's rapid development capabilities and wide range of libraries make it well suited for prototyping ideas and rapidly implementing projects.
- Web Scraping: Python provides powerful libraries such as BeautifulSoup and Scrapy that simplify extracting data from websites and web scraping.
This list only gives an overview of the uses of Java and Python and is not exhaustive. Both languages are versatile and can be used in a variety of application areas, depending on the requirements and goals of a project.
Community und Support
Java and Python both have large and vibrant developer communities, but there are differences in their size and focus.
Java has had an established and extensive community for many years. It is used by a wide range of businesses and organizations and is one of the most widely used programming languages in the world. The Java community is strong in areas such as enterprise applications, server development, large systems, and financial services. There are a number of Java developer conferences, online forums, blogs and forums dedicated to the Java community. The community also offers a large number of open source libraries, frameworks and tools that are developed and maintained by the community itself.
Python has seen an exponentially growing community in recent years. It is particularly popular in areas such as data science, artificial intelligence (AI), machine learning, web development, and automation. Python is characterized by a friendly and welcoming community that places strong emphasis on collaboration and knowledge sharing. There are numerous Python conferences, local meetups, online communities, and forums dedicated exclusively to Python. The Python community has also produced an impressive number of open source projects, libraries, and frameworks that facilitate development in Python.
It is important to note that the size and quality of a community can be subjective and depends on several factors, such as geographic location, industry, projects, and individual developer needs. Both the Java and Python communities provide ample resources, support, and expertise to help developers grow their skills and succeed in their respective application areas.
Future prospects
Both Java and Python have promising futures and are expected to continue to play a significant role in software development. Here are some factors that point to the future prospects of both languages:
Java:
- Stability and distribution: Java has been an established programming language for many years and has proven itself in various industries and application areas. It is supported by a large developer community and used by numerous companies worldwide. The stability and spread of Java indicate that it will continue to play an important role in the future.
- Java as the foundation for other technologies: Java is the foundation for many other technologies and frameworks, including the Android operating system, the Java Virtual Machine (JVM) ecosystem, and various enterprise frameworks such as Spring and Hibernate. Through its role as the foundation for these technologies, Java will continue to have a significant presence in software development.
- Continued development: The Java community is active and engaged and is continuously working to enhance the language. Oracle, the primary custodian of Java, is committed to continuing to support and improve the language and ecosystem. New versions of Java are released regularly to improve functionality and address current development needs.
Python:
- Growing in popularity: Python has gained significant popularity in recent years, especially in areas such as data analytics, artificial intelligence, machine learning, and web development. The increase in demand for these applications indicates that Python will continue to be in demand in the future.
- Simple and more readable syntax: Python has earned a reputation for its simple and readable syntax, making it a popular language for beginners and experienced developers. These features have helped make Python popular as an entry-level programming language and as a tool for rapid prototyping.
- Extensive libraries and frameworks: Python has a large number of libraries and frameworks developed for specific use cases. Libraries such as NumPy, Pandas, TensorFlow, and Django facilitate development in various domains. The availability of these resources contributes to the appeal and popularity of Python.
It is important to note that the technology landscape is evolving rapidly and new languages and tools may emerge. Nevertheless, current trends and widespread use indicate that both Java and Python will play a relevant role in software development in the foreseeable future.