PHP syntax - dream or nightmare
Published
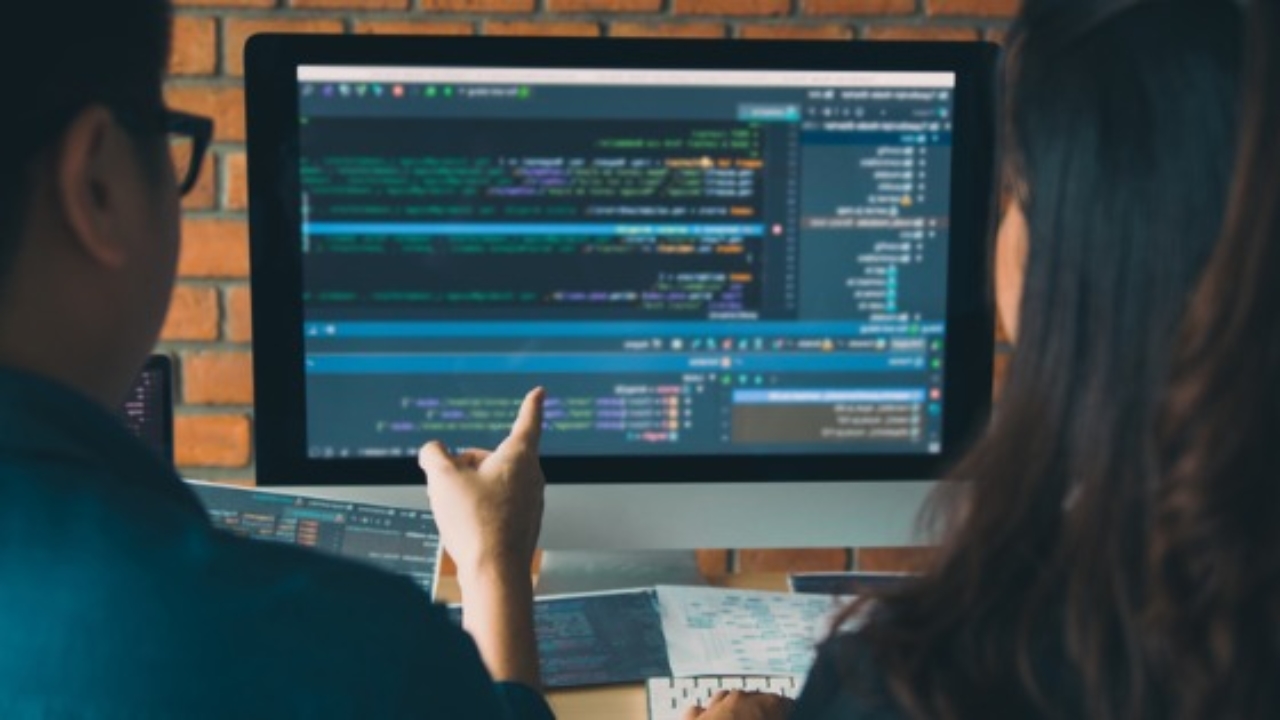
As a software engineer, it is important to become familiar with different programming languages. One of these languages is PHP, which is widely used in web development. In this blog, we will take a closer look at PHP and why it is important for software engineers to master this language.
If you want to refresh your PHP knowledge or even further expand it, we recommend you Skillshare as a learning platform.
Basics of PHP
Variables and data types
PHP is a server-side scripting language primarily used for developing web applications. One of the fundamental concepts in PHP are variables. A variable is a container that stores a value that can be changed during script execution.
To declare a variable in PHP, use the dollar sign ($) followed by a name for the variable. The value of the variable is assigned using the assignment operator (=). PHP supports various data types including:
- Integer: Integers like 1, 2, 3 etc.
- Float: Decimal numbers like 1.2, 3.4, 5.6 etc.
- String: Text like "Hello world", "PHP is great", etc.
- Boolean: Truth values such as true or false.
- Array: A collection of values.
- Object: An instance of a class.
- NULL: A variable with no value.
Operators
Operators are symbols used to perform operations on variables and values. PHP supports various types of operators including:
- Arithmetic operators: +, -, *, /, %
- Comparison operators: ==, !=, <, >, <=, >=
- Logical operators: &&, ||, !
- Assignment operators: =, +=, -=, *=, /=, %=
- Increment and decrement operators: ++, --
- String operations: .
Control structures
Control structures allow you to control the flow of the script by using conditions and loops. PHP supports various types of control structures, including:
- If Statements: Use if statements to execute code when a condition is met.
- Switch Statements: Use switch statements to execute code based on the value of a variable.
- For Loops: Use For loops to execute code a specific number of times.
- While Loops: Use while loops to execute code as long as a condition is met.
- Do-While Loops: Use do-while loops to execute code at least once before the condition is checked.
- Foreach Loops: Use Foreach loops to execute code on each element in an array.
Advanced PHP concepts
Features
PHP functions are an important part of the language and allow developers to organize code and make it reusable. A function is basically a block of code that performs a specific task. You can accept parameters and return values.
There are two types of functions in PHP: user-defined functions and predefined functions. Custom functions are created by the developer and can be used for specific tasks. Predefined functions are already present in PHP and can be used directly.
An example of a custom function:
function addNumbers($a, $b) { return $a + $b; }
This function takes two parameters ($a and $b) and returns the sum of the two.
Classes and objects
Classes and objects are another advanced concept in PHP. A class is essentially a blueprint for an object. It defines the properties and methods that the object will have. An object is an instance of a class.
An example of a class:
class Car{ public $color; public $make; public $model; public function start() { // Code to start the car } public function stop() { // Code to stop the car } }
This class defines a Car object with Color, Make and Model properties and Start and Stop methods.
An example of creating an object:
$myCar = new Car(); $myCar->color = "red"; $myCar->make = "Toyota"; $myCar->model = "Corolla";
This code segment creates a new Car object and assigns it the Color, Make and Model properties.
Inheritance
Inheritance is a concept in object-oriented programming that allows one class to be created based on another class. The new class inherits all properties and methods of the original class and can extend or override them.
An example of inheritance:
class Animal { public $name; public $species; public function eat() { // Code to make the animal eat } } class Dog extends Animal { public function bark() { // Code for the dog to bark } }
This code segment defines an Animal class with the Name property, Species property and the Food method. It also defines a Dog class that inherits from the Animal class and adds a Barking method.
An example of creating a Dog object:
$myDog = new Dog(); $myDog->name = "Fido"; $myDog->species = "Labrador";
This code segment creates a new Dog object and assigns it the Name and Species properties. It also inherits the Eat method from the Animal class.
Working with databases in PHP
PHP is a server-side scripting language commonly used for developing web applications. One of the most important functions of web applications is storing and managing data. In this blog post we will focus on how to work with databases in PHP.
Connect to a database
Before we can start working on a database, we need to connect to it. To do this we need the following information:
- Hostname
- User name
- password
- Database name
We can connect to a database using the function mysqli_connect()
produce. Here is an example:
$hostname = "localhost"; $username = "my username"; $password = "my_password"; $database = "my_database"; $conn = mysqli_connect($hostname, $username, $password, $database); if (!$conn) { die("Connection failed: " . mysqli_connect_error()); }
In this example, we have connected to a database with hostname "localhost", username "my_username", password "my_password", and database name "my_database". If the connection fails, an error message is displayed.
Query data
Once we have connected to a database, we can query data from the database. For this we use the function mysqli_query()
. Here is an example:
$sql = "SELECT * FROM benutzer";$result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { while($row = mysqli_fetch_assoc($result)) { echo "Name: " . $row["name"] . " - E-Mail: " . $row["email"] . ""; } } else { echo "No results found."; }
In this example, we queried all users from the users table and looped through the results. For each user we have output the name and email address.
Changing data
We can also change data in the database. To do this we use the functions mysqli_query()
and mysqli_affected_rows()
. Here is an example:
$sql = "UPDATE benutzer SET name='Max Mustermann' WHERE id=1"; $result = mysqli_query($conn, $sql); if (mysqli_affected_rows($conn) > 0) { echo "Record updated successfully."; } else { echo "No records updated."; }
In this example, we changed the name of the user with ID 1 in the users table to John Doe. If the change was successful, a success message is displayed. Otherwise an error message will be issued.
Web development with PHP
Basics of web development
Web development is a broad field that deals with the creation of websites and web applications. PHP is one of the most commonly used programming languages for web development. It is a server-side scripting language used for creating dynamic web pages.
To start web development with PHP, you need a basic knowledge of HTML, CSS, and JavaScript. HTML is used to define the structure of the web page, CSS is used to design the look of the web page, and JavaScript is used to implement the interactivity of the web page.
PHP runs on the server and generates HTML code that is sent to the user's browser. PHP can also interact with databases such as MySQL or PostgreSQL to generate dynamic content on the website.
Working with forms
Forms are an important part of web pages because they allow users to submit data to the server. PHP can be used to process and validate form data.
To create a form in HTML you need to