Java Lambda and Functional Programming: An Introduction
Published
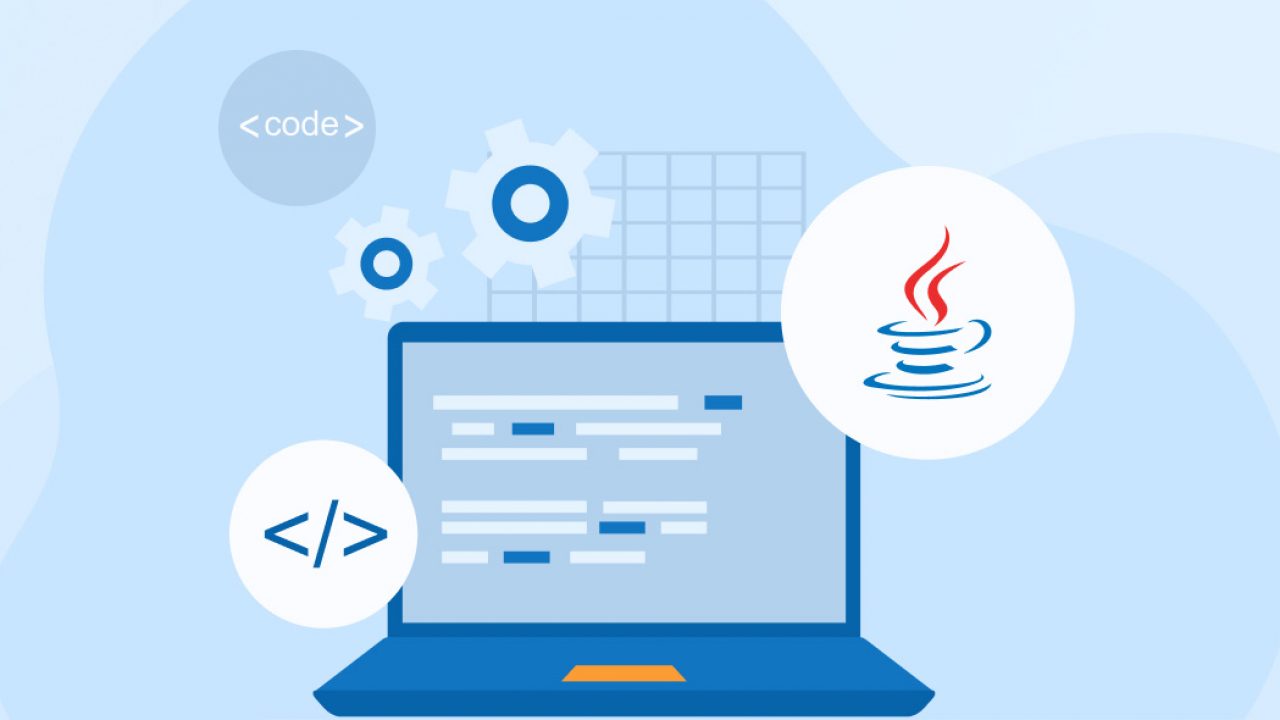
The basics of lambda expressions in Java
Lambda expressions are one of the most exciting additions to the world of Java programming, introduced with Java 8. They provide a compact way to pass functions as arguments to other functions or even create them directly in the code without the need to write a separate method. A lambda expression consists of a parameter list, an arrow operator "->" and an expression. These expressions can be applied to functional interfaces that have only one abstract method. A basic example of a lambda expression looks like this: (int a, int b) -> a + b
A lambda expression is defined here that adds two integers. The expression itself is "a + b", and the parameter list contains the two integers to be added. Lambda expressions allow for a shorter and more readable syntax, especially when you pass functions as arguments to high-order functions such as map
, filter
or reduce
transfer. They make working with collections and stream APIs much easier and promote writing code with higher abstraction and less boilerplate. In the coming sections, we will dive deeper into the world of lambda expressions in Java and explore their various applications and possibilities.
Lambda expressions vs. anonymous inner classes: The readability revolution
Lambda expressions have triggered a revolution in the Java world in terms of code readability and writability, especially when compared to anonymous inner classes. In the past, developers often had to use anonymous inner classes to attach functionality to existing classes or interfaces. This resulted in a lot of boilerplate code that affected the readability of the code. A lambda expression, on the other hand, is extremely compact and focuses on the essentials. It can often reduce the entire code of an anonymous inner class to one line. For example, while an anonymous inner class for sorting a list by name might look like this: Collections.sort(names, new Comparator
Collections.sort(names, (a, b) -> a.compareTo(b));
Lambda expressions not only eliminate the superfluous code, but they also make the code much easier to understand by focusing on the developer's intent and avoiding unnecessary abstractions. This has greatly improved the readability and maintainability of Java code and allows developers to focus on what they want their programs to do, rather than the code they need to write to achieve it. In the coming sections, we will dive further into the details of lambda expressions and see how they can be used in different situations in Java development.
Functional interfaces: The building blocks for lambda expressions
Functional interfaces are at the heart of lambda expressions in Java. They form the basis on which lambda expressions are created and used. A functional interface is an interface with only one abstract method. This means that it defines exactly one method that must be implemented, and often this is a method that describes a specific function. A classic example of a functional interface in Java is the "Runnable" interface, which defines a single method called "run()". Here is a simple example of how a lambda expression can be used to start a thread with a runnable interface: Runnable myRunnable = () -> { // Here comes the code for the thread's task }; Thread thread = new Thread(myRunnable); thread.start();
Lambda expressions are particularly useful when used in conjunction with functional interfaces, as they greatly simplify the implementation of these interfaces. Developers can focus on the specific task that the functional interface describes without having to worry about the details of an anonymous inner class. It is important to note that functional interfaces can also be marked in Java as the annotation "@FunctionalInterface". This signals to the compiler that this interface is intended for lambda expressions and should only contain an abstract method. The use of functional interfaces and lambda expressions allows Java developers to write efficient and readable code that clearly expresses the intentions behind the functions. In the following sections, we will explore more functional interfaces in Java and see how they can be used in different applications.
Practical applications of Java Lambda in modern development
Java lambda expressions have proven to be extremely useful in modern software development and are used in a variety of use cases. One of the most common use cases is working with lists and collections, where lambda expressions are used to perform complex operations on the elements. Here is an example of filtering elements in a list with lambda expressions: Cunning
ExecutorService executor = Executors.newFixedThreadPool(4); executor.submit(() -> { // Here comes the task for the first thread }); executor.submit(() -> { // Here comes the task for the second thread });
Lambda expressions also make it easier to work with Swing and JavaFX, where they can be used to write event-driven code for graphical user interfaces. They allow developers to create responsive user interfaces by defining event handlers in a compact and readable way. In addition to these examples, there are many other uses for lambda expressions in modern Java development, including processing streams, implementing design patterns such as the Strategy pattern, and much more. Their ability to simplify code and increase readability makes them a valuable tool for developers in today's software development.