Python Lambda Functions: An introduction
Published
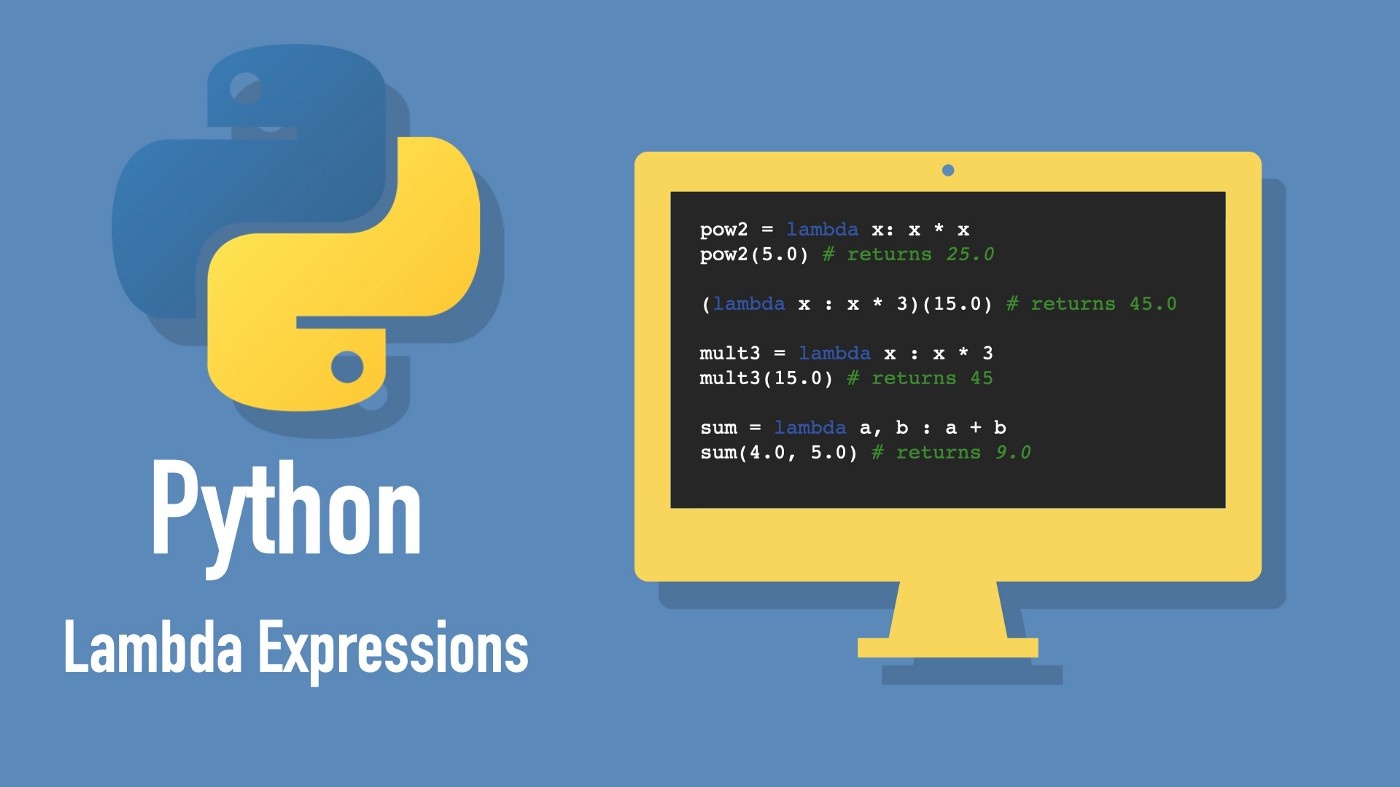
Basics of lambda functions in Python
Lambda functions, also known as anonymous functions, are a powerful concept in Python. They allow you to create small, single-use functions without using the "def" keyword. Here are some basic concepts you should know about lambda functions in Python:
- Definition: A lambda function in Python is created with the keyword "lambda", followed by parameters and an expression. For example:
lambda x: x * 2
. This function multiplies the parameter "x" by 2 and returns the result. - Simple syntax: Lambda functions are suitable for simple operations and have a compact syntax. They are particularly useful if you need a function that is only used once and does not need to be named.
- Use: Lambda functions are often used in functions such as
map()
,filter()
andreduce()
are used to apply operations to lists or sequences. They offer a convenient way of defining functions directly on the spot. - Limitations: Lambda functions should be used sparingly as they are limited to simple expressions and cannot contain complex statements. For extensive functions, it is advisable to use named functions.
Lambda functions are a useful tool for simplifying Python code and making it more readable, especially in combination with functions such as map()
and filter()
. In the following subtitles, we will go further in depth to explore the many applications and best practices of using lambda functions in Python.
Anonymous functions: The role of lambda in Python
Lambda functions in Python are anonymous functions, which means that they do not have their own names. This anonymity makes them extremely flexible and practical, especially in situations where you need a small function that is only used once. The role of lambda in Python is to quickly create and use these small, ad-hoc functions.
- Simple creation: With Lambda, you can create functions directly in your code without having to create a separate definition with the keyword "def". This saves time and simplifies the code.
- Inline use: Lambda functions are particularly useful when you need to pass functions as arguments to other functions. For example, you can use them in combination with
map()
to apply an operation to all elements of a list without having to create a separate function. - Readability: Although lambda functions are compact, it is important to ensure that they do not make the code unnecessarily complicated. They should be used sparingly, especially for simple operations, to ensure readability.
- Restrictions: Lambda functions have some restrictions. They can only consist of a single expression and cannot contain complex statements. If you need an extensive function with many statements, it is better to create a named function.
In Python, lambda functions play an important role in implementing higher level functions and providing flexible ways to transform data. In the following sections, we will look at other use cases and best practices for using lambda functions in Python.
Lambda vs. named functions: Advantages and disadvantages
The choice between lambda functions and named functions in Python depends on the requirements of your code and your programming style. Each option has its own advantages and disadvantages that you should consider when making your decision.
- Advantages of lambda functions:
- Compactness: Lambda functions are extremely compact and can be written in one line, which makes the code easier to read, especially for simple operations.
- Anonymity: Since lambda functions do not have their own names, they are perfect for one-off, ad-hoc functions that are used in a specific context and do not require a global name.
- Inline use: You can use lambda functions directly on the spot, e.g. as arguments for functions such as
map()
andfilter()
which simplifies code creation.
- Disadvantages of lambda functions:
- Limited complexity: Lambda functions are limited to simple expressions and cannot contain complex statements or multiple lines of code. This can impair readability if they are used excessively.
- Limited reusability: Since lambda functions are anonymous, they cannot simply be reused. If you want to use a function in different parts of your code, a named function is often more practical.
Named functions, on the other hand, offer more structure and reusability, but are often longer and require a separate definition. It is therefore important to weigh up the pros and cons of the two approaches and choose the one that best suits your particular use case. In the next sections, we will see how you can use lambda functions effectively and when it is better to use named functions.
Practical applications of lambda functions
Lambda functions in Python have a wide range of practical applications that make code creation more efficient and readable. Here are some examples of how lambda functions can be used in practice:
- Transforming lists: With functions such as
map()
andfilter()
lambda functions can be used to transform or filter elements in a list. For example, you can usemap()
apply a lambda function to all elements of a list to create a new list. - Sorting data: Lambda functions can be used as keys in sorting functions such as
sorted()
can be used. They make it possible to sort according to certain criteria without changing the original data. - User-defined sorting: If you need a special sort order, you can create a lambda function that defines the comparison rules to be used by the sorting algorithm.
- Filtering data: With
filter()
and a lambda function, you can remove elements from a list that do not meet certain criteria. - Mathematical calculations: Lambda functions are well suited for simple mathematical calculations or expressions, especially in combination with functions such as
reduce()
.
The use of lambda functions makes it possible to keep the code compact and express specific operations in an easily readable format. However, it is important to use them sparingly and depending on the situation, as they may not be suitable for more complex tasks. The next sections will dive deeper into use cases and provide best practices for using lambda functions in different contexts.