The art of compilation: everything you need to know
Published
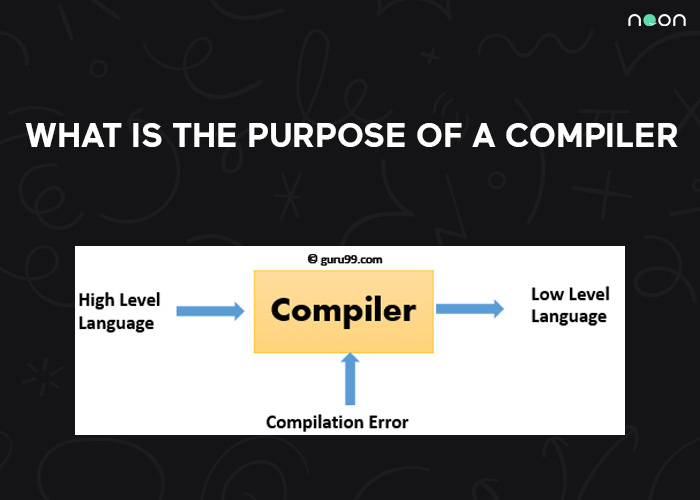
Compilation basics: An introduction
Compilation is an essential process in the world of software development that enables the transition from human-readable source code to executable machine code. It forms the backbone of most modern programming languages and ensures that our applications work on different platforms and systems. During compilation, the source code is analyzed by a compiler and translated into an intermediate representation, often referred to as object code. This object code is then further processed to produce the final machine code that the computer can execute.
The compilation process allows developers to code flexibly in high-level languages such as C++, Java or Python without having to worry about the specific hardware or architecture of a target system. It is this compilation step that ensures the portability of software, as the same source code can be used on different platforms without having to rewrite it. In addition, compilation also offers the advantage of code optimization, allowing programs to run faster and more efficiently.
In the rest of this blog, we will dive deep into the compilation process, explore the different stages of compilation and better understand the importance of compilation in today's software development.
Compilation process in detail: From source code to executable program
The compilation process is a complex procedure that converts the source code into executable machine code. This process can be roughly divided into several phases, which are explained in more detail below:
- Lexical analysis: In this phase, the source code is split into individual tokens. Comments are also removed and line endings are standardized. This step prepares the code for further processing.
- Syntaxanalyse: This checks whether the sequence and structure of the tokens correspond to the grammatical rules of the programming language. This step ensures that the code is written correctly.
- Semantic analysis: In this phase, the source code is checked for semantic errors, e.g. whether variables are declared but not used. This analysis ensures that the code makes sense.
- Code generation: The compiler creates the intermediate code, also known as object code, from the analyzed and checked source code files. This object code is platform-independent and contains instructions that can be executed by the target machine.
- Linking: If a program consists of several source code files, these are linked in this phase to form a single executable program. Libraries and external modules are also integrated.
- Optimization: Optionally, but crucial for performance, the compiler can optimize the generated code. This means that the object code is rewritten in such a way that it is executed faster or more efficiently.
Once this process is complete, an executable program is created that can be run on the target computer. The compilation process enables developers to convert human-readable source code into machine language and thus create software for different platforms and architectures.
Compilation process in detail: From source code to executable program
The compilation process is a crucial step in software development in which the human-readable source code is converted into executable machine instructions. This process can be roughly divided into several successive phases, which must be considered in detail:
- Lexical analysis: This phase is the first step of the compilation process. The source code is split into individual lexemes or tokens, with spaces and line breaks removed. This puts the code into a form that the compiler can understand.
- Syntaxanalyse: In this phase, the grammar of the source code is checked. The compiler ensures that the arrangement of the tokens corresponds to the syntactic rules of the programming language. If errors or invalidities are detected, an error message is generated.
- Semantic analysis: Here, the code is checked for semantic errors. This means that the compiler ensures that variables are declared and used correctly and that all references to functions and objects make sense.
- Code generation: In this phase, the source code is translated into intermediate code or so-called object code. This intermediate code is platform-independent and contains instructions that are understood by the target machine.
- Optimization: If necessary, compilers can also optimize the generated code. This includes, for example, the removal of unused variables or the conversion of instructions to improve performance.
- Linking: If a program consists of several source code files or accesses external libraries or modules, these are linked together in this phase. This creates a complete executable program.
Once these phases have been completed, an executable program is created that can be run on the target computer. The compilation process is essential for the development of software as it converts the source code into a form that is understood by the hardware. This enables developers to create programs for different platforms and architectures.
Compilation troubleshooting: common problems and solutions
Compiling source code is a complex process that is often associated with various challenges and errors. Here are some common problems that can occur during compilation and possible solutions:
- Syntax error: These are errors where the syntax rules of the programming language are not adhered to. The compiler normally issues an error message indicating the line in question in the source code. The solution is to adapt the code according to the syntax rules.
- Semantic error: Semantic errors occur when the code is syntactically correct but still functions incorrectly. This can be due to incorrect variable assignments, invalid calculations or incorrect use of functions. These errors often require a thorough review of the code in order to find and rectify the cause.
- Undefined references: If variables or functions that have not been previously defined are accessed in the code, an error occurs. The solution is to ensure that all references point to correct declarations and that the correct header files or libraries are included.
- Compatibility problems: Compatibility problems can occur when compiling code on different platforms or with different compiler versions. This often requires adjustments to the code or the use of platform-specific directives.
- Memory leaks: Memory leaks occur when memory is allocated dynamically in the code but is not released properly. This can lead to unwanted memory consumption and affect the stability of the program. Memory leaks must be eliminated through careful management of memory allocations.
Troubleshooting during compilation often requires patience and thorough knowledge of the programming language and compiler behavior. The ability to identify problems and find effective solutions is crucial for developers to create high-quality and reliable software.