Java vs C# - Which language is better?
Published
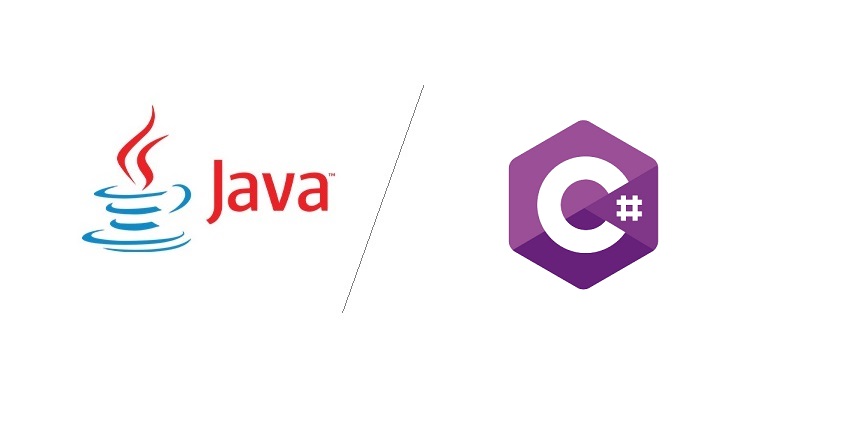
Java and C# are two of the most popular programming languages in software development. Both languages have a strong foundation in object-oriented programming and are used in various fields, from web development to enterprise software and game development.
If you want to refresh your Java/C# knowledge or even extend it further, we recommend Skillshare as a learning platform.
Although Java and C# have many similarities, there are also some differences in terms of syntax, platform support, frameworks, and application domains. In this comparison we will take a deeper look into Java and C# gain a better understanding of their strengths, application areas, and differences. Whether you're an experienced developer looking to learn a new language or are faced with deciding which language is right for your next project, this comparison of Java and C# will help you make an informed decision.
Aspect | Java | C# |
---|---|---|
Developer company | Oracle Corporation | Microsoft Corporation |
Year of publication | 1995 | 2000 |
Typing | Static | Static |
Platform | Independent (Java Virtual Machine - JVM) | Mainly for Windows (Common Language Runtime - CLR) |
Syntax | Similar to C/C++ | Similar to Java |
Scope | Web Apps, Android Apps, Enterprise Software, Big Data, IoT | Windows applications, web applications, game development, enterprise software |
Frameworks | Spring, Hibernate, JavaFX, Apache Struts, JSF | .NET Framework, ASP.NET, Entity Framework, Windows Presentation Foundation (WPF) |
Multi-Paradigm | Supports object-oriented programming, concurrent programming and functional programming | Supports object-oriented programming, concurrent programming and functional programming |
Portability | High portability through the Java Virtual Machine (JVM) | Main platform is Windows, but there are also implementations for other platforms like Mono and .NET Core |
Performance | Usually slightly faster than C# | Usually somewhat slower than Java |
Popularity | Widely used and popular for web and Android development | Popular for Windows and .NET development |
It is important to note that Java and C# offer similar features and concepts, as both are based on C/C++ syntax and are strong object-oriented programming languages. The choice between Java and C# depends on the specific requirements of the project, the target platform and the personal preferences of the developer. Both languages have a large developer community and a wide range of tools and frameworks to facilitate the development of software solutions.
Basics
Java and C# are two popular programming languages that share many similarities, as they are based on the syntax of C/C++ and are strong object-oriented languages. Nevertheless, there are some important differences between the two languages:
Difference | Java | C# |
---|---|---|
Platform dependency | Platform independent (Java Virtual Machine - JVM) | Mainly for Windows (Common Language Runtime - CLR) |
Development environment | Eclipse, IntelliJ IDEA | Microsoft Visual Studio |
Event handling | Implementation of listener interfaces | Use of events and delegates |
Nullable Types | Not supported | Supported with Nullable Types |
Exceptions | Checked Exceptions | Unchecked Exceptions |
Namespaces | Use of packages | Use of namespaces |
Lambda expressions | Available from Java 8 | Available |
Extension methods | Not supported | Supports extension methods |
Threads and concurrency | Extensive thread support | Task Parallelism, Parallel Extension Library |
- Platform dependency: Java was designed from the beginning to be a platform-independent language. Java code is compiled into bytecode that is executed by a Java Virtual Machine (JVM), which means that Java applications can run on different operating systems as long as a compatible JVM is available. C#, on the other hand, was designed primarily for development on Microsoft Windows, although there are implementations such as Mono and .NET Core that allow cross-platform development.
- Ecosystem and frameworks: Java has an extensive ecosystem and a large number of frameworks, libraries and tools suitable for various application areas such as web development (e.g. Spring, Hibernate), Android development (Android SDK) and Big Data (Apache Hadoop). C# is based on the .NET Framework or platform, which provides a variety of frameworks and tools for Windows applications (e.g. Windows Presentation Foundation - WPF, Windows Forms), web applications (ASP.NET) and game development (Unity Engine).
- Performance: There are differences between Java and C# when it comes to performance. In general, Java is slightly faster than C# because it inherently offers efficient code optimization techniques. However, C# also offers good performance and is particularly well optimized for Windows application development.
- Syntax: Although both languages are based on C/C++, they also have some syntactical differences. Java's syntax is more C-like, while C#'s syntax is more Java-like. For example, Java uses the "extends" keyword for inheritance, while C# uses the "inherits" keyword.
- Enterprise environment: C# has strong integration with the Microsoft development platform and is widely used in enterprises that use Microsoft technologies. Java, on the other hand, is widely used in various enterprise environments and is also used for large web applications and enterprise software.
- Development environments: Java is often developed using the Eclipse or IntelliJ IDEA development environment, while C# is usually developed using Microsoft Visual Studio. Each development environment offers specific functions and tools tailored to the respective language and platform.
- Event handling: In Java, event handling is achieved by implementing listener interfaces and overriding callback methods. C# uses the concept of events and delegates, which provides a more flexible and intuitive way of event handling.
- Nullable Types: C# supports nullable types, which allow variables to take on an additional null value. In Java, all reference types are implicitly nullable unless they are initialized with the null keyword.
- Exceptions: Java uses checked exceptions, where the Compiler ensures that exceptions are handled or declared. C#, on the other hand, uses unchecked exceptions where no explicit handling or declaration is required.
- Namespaces: In Java, packages are used to organize classes and avoid collisions between classes with similar names. C# uses namespaces to serve similar purposes.
- Lambda expressions: C# provides support for lambda expressions, which provide a compact notation for anonymous functions. In Java, lambda expressions have only been available since the introduction of Java 8.
- Extension methods: C# allows the creation of extension methods that can be used to add new methods to existing classes without having to modify them. Java does not support direct creation of extension methods.
- Threads and Concurrency: Java has rich support for threads and concurrency and provides an extensive API for working with threads. C# has similar features, but with the difference that it provides Task Parallelism and the Parallel Extension Library, which allow easier handling of concurrency.
It is important to note that Java and C# offer similar concepts and features, and many developers find it relatively easy to switch between the two languages. The choice between Java and C# depends on the specific requirements of the project, the target platform, and the existing skills and preferences of the development team. Both languages are popular, well documented, and have a large developer community to assist in the development of high-quality software.
Syntax
Syntax differences | Java | C# |
---|---|---|
Class and method declaration | public class MyClass { ... } | public class MyClass { ... } |
Inheritance | class ChildClass extends ParentClass | class ChildClass : ParentClass |
Interface implementation | class MyClass implements MyInterface | class MyClass : MyInterface |
Static methods and fields | public static void myMethod() { ... } | public static void MyMethod() { ... } |
Namespaces | package com.example; | using System; |
Import classes and packages | import com.example.MyClass; | using System; |
Exception handling | try { ... } catch (Exception e) { ... } | try { ... } catch (Exception e) { ... } |
String concatenation | String result = "Hello, " + name; | string result = "Hello, " + name; |
Grinding | for (int i = 0; i < 10; i++) { ... } | for (int i = 0; i < 10; i++) { ... } |
Conditional instructions | if (condition) { ... } else { ... } | if (condition) { ... } else { ... } |
Switch statements | switch (variable) { ... } | switch (variable) { ... } |
Enumerationen | enum MyEnum { VALUE1, VALUE2, ... } | enum MyEnum { VALUE1, VALUE2, ... } |
Both Java and C# have a similar abstract syntax, since both are based on the syntax of C/C++ and are strong object-oriented languages. However, there are some differences in syntax that can be subjectively perceived as more abstract or less abstract.
Java has a more C-like syntax that can be considered relatively simple and readable. The use of curly braces for block structuring and the "extends" keyword for inheritance are some examples of Java syntax.
C#, on the other hand, has a similar syntax to Java, but with some differences. Some developers find the syntax of C# to be a bit more abstract because it has additional concepts and syntactic sugar types. Examples include lambda expressions, the use of "var" for implicit type inference, the concept of properties instead of getter and setter methods, and support for extension methods.
It is important to note that the perception of the abstractness of the syntax is subjective and depends on the individual experience and preferences of the developers. Both languages provide a clear and structured syntax that allows developers to write readable and maintainable code. Ultimately, the choice of language should depend not only on the syntax, but also on other factors such as the target platform, the available frameworks and tools, and the requirements of the project.
Platform independence
The platform independence between Java and C# differs in their approach and availability.
Java was designed from the beginning to be a platform-independent language. Java code is compiled into bytecode that is executed by a Java Virtual Machine (JVM). This allows Java applications to run on different operating systems as long as a compatible JVM is available. The JVM acts as a kind of "translator" between the platform-independent bytecode and the operating system. This concept allows developers to write Java applications once and run them on different platforms, such as Windows, macOS and Linux. Java's platform independence has contributed to its widespread use in various fields, including web applications, mobile development, and enterprise software.
C#, on the other hand, was primarily designed for development on Microsoft Windows. The official implementation of C# and the .NET Framework is closely tied to the Windows operating system environment. C# applications are based on the Common Language Runtime (CLR), which is specific to Windows. This means that C# applications typically run smoothly on Windows platforms, such as Windows desktops and Windows Server. However, Microsoft has also developed implementations such as Mono and .NET Core to enable cross-platform C# development. With Mono and .NET Core, C# applications can run on other operating systems such as macOS and Linux. These implementations provide some platform independence for C#, although full support and integration with the Windows platform may not.
It is important to note that platform-independent development in C# with Mono and .NET Core may require additional effort to ensure compatibility with different platforms. When choosing between Java and C# for platform-independent development, it is advisable to consider the specific requirements of the project, the tools and frameworks available, and the target platforms. Java inherently offers greater platform independence and is therefore often the preferred choice for platform-independent development.
Object orientation
Both Java and C# are strongly object-oriented programming languages and support the basic principles of object orientation such as encapsulation, inheritance, and polymorphism. However, there are some differences in the way object orientation is implemented in Java and C#. Here are some important aspects of object orientation in both languages:
- Classes and objects: In Java and C#, classes are used to create objects. A class defines the properties (attributes) and behavior (methods) of an object. Both languages support the definition of classes and the creation of objects in a similar way.
- Inheritance: Both languages support inheritance, where a class can be derived from another class. Java uses the "extends" keyword to derive a class from another class, while C# uses the colon symbol ":".
- Interfaces: Java and C# support the implementation of interfaces. An interface defines a contract that a class must fulfill in order to implement certain methods. Java uses the keyword "implements" to implement an interface, while C# uses the colon symbol ":".
- Access modifiers: Both languages provide access modifiers to control access to classes, methods, and attributes. Examples of access modifiers are "public", "private" and "protected".
- Polymorphism: Java and C# support polymorphism, where an object of a given class can be treated as an object of another class if they are derived from a common superclass. This is achieved through inheritance and method overrides.
- Abstract classes: Both languages allow the definition of abstract classes that serve as templates for other classes and cannot be instantiated directly.
It is important to note that both Java and C# have continuously evolved and introduced new features and improvements in object orientation. However, the basic concepts of object orientation are present in both languages. The choice between Java and C# for object-oriented programming depends on the specific requirements of the project, the existing skills of the development team, and other factors.
Purpose
Here is a table summarizing some of the common uses of Java and C#:
Intended use | Java | C# |
---|---|---|
Web applications | Java EE Platform, Servlets, JSP, Spring | ASP.NET, ASP.NET Core, MVC-Frameworks |
Mobile App Development | Android development (Java, Kotlin) | Xamarin, Unity (for games) |
Enterprise Software | Java EE platform, Spring, Hibernate | .NET-Framework, Entity Framework |
Big Data processing | Hadoop, Spark, Apache Kafka | Hadoop, Spark, Apache Kafka |
Internet of Things (IoT) | Java Embedded, MQTT, Eclipse IoT Framework | .NET Micro Framework, Windows IoT Core |
Game development | JavaFX, LibGDX, jMonkeyEngine | Unity, MonoGame, Unreal Engine (with C# support) |
Desktop applications | JavaFX, Swing | Windows Forms, WPF, UWP |
Finance | Java SE, Java EE, Spring | .NET-Framework, ASP.NET |
Scientific calculations | Java SE, Apache Commons Math, Weka, JAMA | .NET-Framework, Math.NET Numerics |
Database applications | JDBC, JPA (Java Persistence API), Hibernate | ADO.NET, Entity Framework |
Performance
Benchmarking the performance of programming languages such as Java and C# can depend on many factors, including the specific implementation, hardware, compiler optimizations, and other variables. It is important to note that benchmarks only provide a snapshot of performance and actual results may vary depending on the scenario. Here is a general table that can provide an overview of Java and C# performance:
Aspect | Java | C# |
---|---|---|
Runtime performance | Usually slightly faster than C# | Usually somewhat slower than Java |
Memory consumption | Moderate memory utilization | Moderate memory utilization |
Garbage Collection | Uses generational GC (e.g. G1 GC) | Uses generational GC (e.g. CLR GC) |
Just-in-time compilation | HotSpot JVM compiles code at runtime | .NET JIT compiler compiles code at runtime |
Parallelism | Supports multi-thread and concurrency programming | Supports multi-thread and concurrency programming |
Mathematical operations | Good performance for numerical operations | Good performance for numerical operations |
Startup Time | Moderate startup time | Moderate startup time |
Code optimization | Extensive code optimization options | Extensive code optimization options |