Effective database management with Java Hibernate: A look at the powerful ORM framework
Published
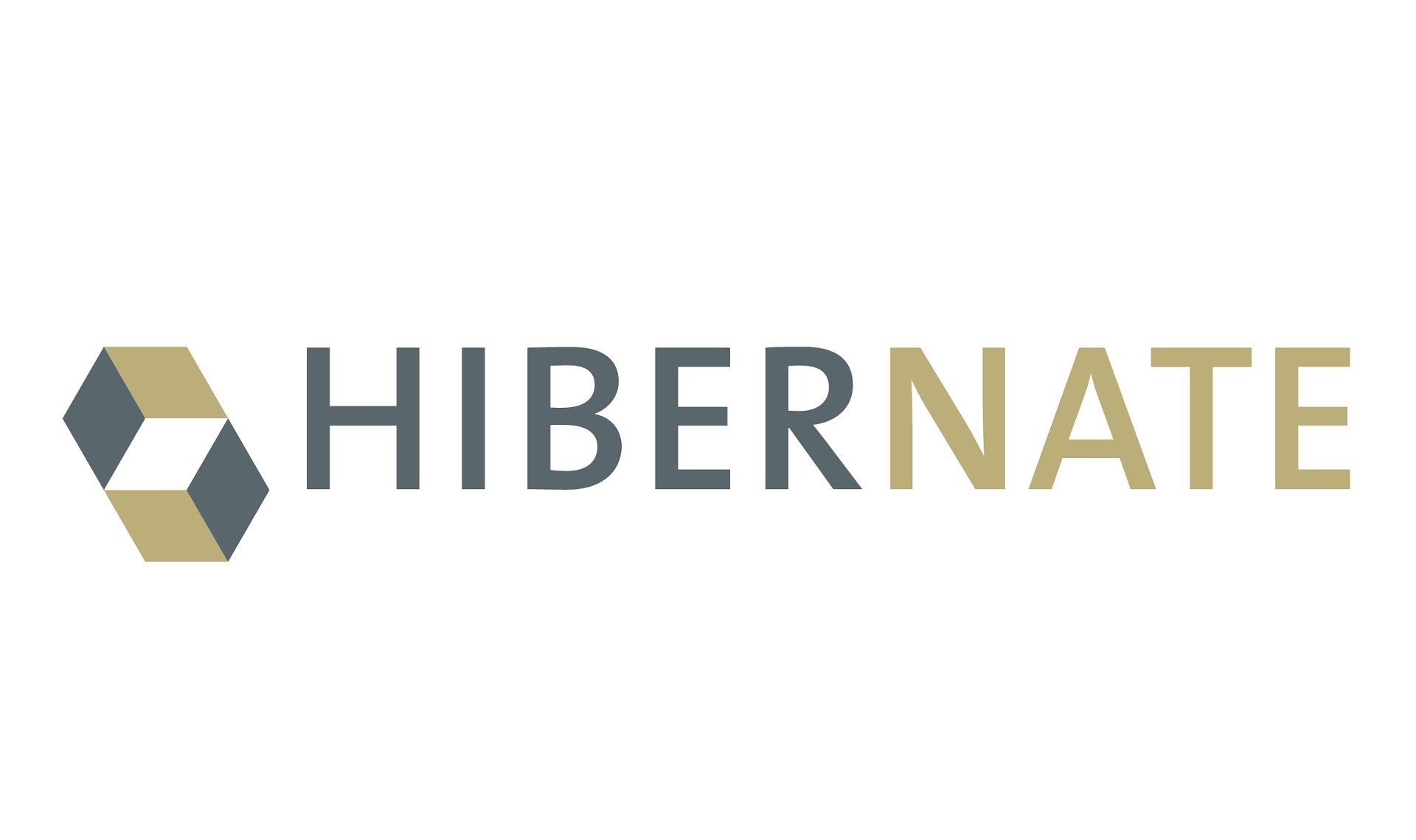
What is Hibernate?
Hibernate is an open source framework for the Java platform which facilitates the development of database applications. It provides an object-oriented interface to the database that enables developers to integrate database queries into Java code. Hibernate is an ORM framework (Object-Relational Mapping) that maps the relationship between objects in Java and tables in a relational database.
If you want to refresh or even expand your Java knowledge, we recommend Skillshare as a learning platform.
Hibernate | Description |
---|---|
ORM-Framework | Java Hibernate is a powerful ORM framework for object-relational mapping of databases. |
Database independence | Hibernate enables platform-independent development, as it can work with various relational databases. |
Object-relational mapping (ORM) | Hibernate enables the seamless mapping of Java objects to relational database tables and vice versa. |
Simple database operations | Hibernate simplifies the creation, reading, updating and deletion (CRUD) of database data through its APIs and query languages such as HQL and Criteria API. |
Transaction management | Hibernate supports transaction management so that database operations can be executed in atomic transactions. |
Caching mechanisms | Hibernate offers various caching options to optimize performance by caching frequently accessed data. |
Lazy Loading | Hibernate supports lazy loading, in which associated objects are only loaded from the database when required in order to improve performance. |
Support for mapping annotations | Hibernate supports annotations to specify the mapping of Java classes to database tables and to simplify configuration. |
Integration with JPA | Hibernate implements the Java Persistence API (JPA) and can be seamlessly integrated with other JPA-compatible frameworks and tools. |
Aktive Community und Support | Hibernate has a large and active community that provides extensive documentation, tutorials and support. |
History
Hibernate was first developed in 2001 by Gavin King and has since become one of the most popular frameworks for Java development. It is part of the JBoss project and is supported by Red Hat.
Advantages
Hibernate offers many advantages for the development of database applications, including:
- Object-oriented interface to the database
- Reduction of boilerplate code
- Support for transactions and caching
- Support for various databases
- Increased productivity through automatic mapping of objects to tables
Installation of Hibernate
System requirements
Hibernate requires Java 8 or higher and a supported database such as MySQL , Oracle or PostgreSQL .
Download und Installation
Hibernate can be downloaded from the official website. Once downloaded, it can be integrated into the project by copying the Hibernate libraries into the project's classpath directory.
Konfiguration
Hibernate must be configured in order to communicate with the database. The configuration takes place in an XML file that contains the connection information to the database. This file must be available in the class path of the project.
First steps with Hibernate
Strengths of Hibernate | Weaknesses of Hibernate |
---|---|
Powerful ORM framework | Complexity and steep learning curve for beginners |
Simplification of database interaction | Configuration effort and complex mapping for complex data structures |
Platform independence and support for various databases | Potentially increased resource consumption and overhead |
Efficient transaction management | Potential impact on performance with insufficient optimization |
Support for caching mechanisms | Difficulties in troubleshooting and diagnosing errors |
Lazy loading to improve performance | Challenges in the treatment of complex associations |
Integration with JPA and other frameworks | Restrictions on flexibility and adaptability |
Active community and extensive resources | Requires good knowledge of relational database concepts |
Creating a database
Before Hibernate can be used, a database must be created. This can be done with a database administration tool such as MySQL Workbench or phpMyAdmin.
Creating entity classes
Entity classes are Java classes that represent the database tables. Each entity class must be marked with the @Entity annotation.
Creating Hibernate configuration files
The Hibernate configuration file contains the connection information to the database as well as other settings such as caching and transaction management.
Creating DAO classes
DAO classes (Data Access Object) are Java classes that execute database queries. They use Hibernate to retrieve, update or delete data from the database.
Creating test classes
Test classes are used to test the functionality of the DAO classes. You can use JUnit tests or other test frameworks.
Mapping in Hibernate
Object-Relational Mapping (ORM)
ORM is the process of mapping objects in Java to tables in a relational database. Hibernate uses ORM to define the relationship between entity classes and database tables.
Mapping of entity classes to database tables
Each entity class is assigned to a database table. The @Table annotation is used to define the table to which the entity class is mapped.
Mapping of attributes to columns
Each attribute in an entity class is assigned to a column in the associated database table. The @Column annotation is used to define the column to which the attribute is mapped.
Mapping of relationships between entity classes
Hibernate supports different types of relationships between entity classes, including one-to-one, one-to-many and many-to-many. The @OneToOne, @OneToMany and @ManyToMany annotations are used to define the relationships.
Hibernate Query Language (HQL)
Basics of HQL
HQL is an object-oriented query language used by Hibernate to retrieve data from the database. HQL queries are similar to SQL queries, but use objects and attributes instead of tables and columns.
Creating HQL queries
HQL queries are defined in a string variable and can contain parameters that are replaced at runtime. HQL queries can be used in DAO classes to retrieve data from the database.
Use of HQL in DAO classes
DAO classes use HQL queries to retrieve data from the database. The results are converted into Java objects and returned to the caller.
Transactions in Hibernate
Transaction basics
Transactions are used to execute multiple database operations as a single unit. If a transaction fails, all changes are undone.
Use of transactions in DAO classes
DAO classes use transactions to update, delete or add data in the database. Transactions are started and ended in the DAO class.
Transaction management in Hibernate
Hibernate offers various options for transaction management, including the use of JTA (Java Transaction API) or the use of Hibernate transactions.
Optimization of Hibernate
Use of caching
Caching can improve the performance of Hibernate applications by caching frequently accessed data. Hibernate supports different types of caching, including first-level caching and second-level caching.
Optimization of database queries
Database queries can be optimized by creating indexes and avoiding unnecessary queries. Hibernate also offers the possibility to optimize queries by changing the fetch strategy.
Use of lazy loading
Lazy loading is a technique in which data is only retrieved from the database when it is needed. This can improve the performance of Hibernate applications by avoiding unnecessary data queries.